50 Most Commonly Used Python Code Snippets for Everyday Programming
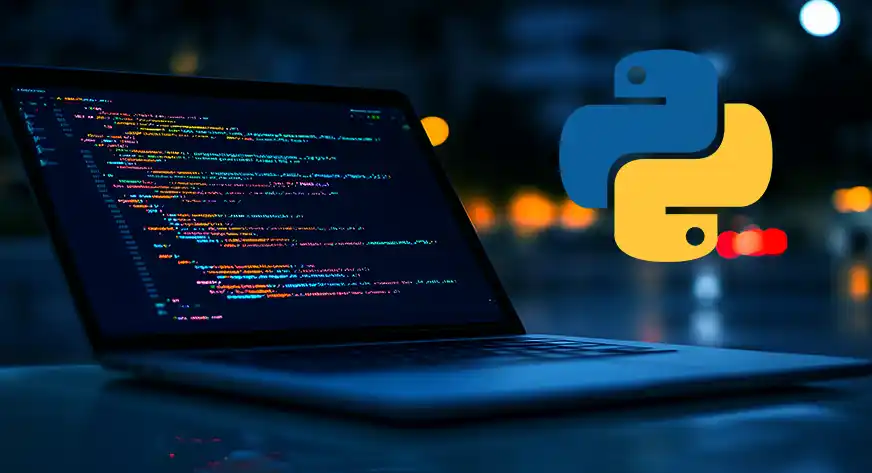
Python, owing to its simplicity and expressiveness, remains one of the most loved programming languages in the world. Whether you are a beginner or a seasoned developer, having a handy set of frequently used code snippets can save considerable time. This article presents 50 widely used Python code snippets, categorised for clarity and practical use.
๐ List Operations
1. List Comprehension with Condition
squares = [x**2 for x in range(10) if x % 2 == 0]
2. Flatten a Nested List
nested = [[1, 2], [3, 4]]
flat = [item for sublist in nested for item in sublist]
3. Remove Duplicates from a List
unique_items = list(set(my_list))
4. Reverse a List
reversed_list = my_list[::-1]
5. Sort List of Dictionaries by Key
sorted_list = sorted(dicts_list, key=lambda x: x['key_name'])
๐ File Handling
6. Read a File
with open('file.txt', 'r') as file:
content = file.read()
7. Write to a File
with open('file.txt', 'w') as file:
file.write('Hello, World!')
8. Append to a File
with open('file.txt', 'a') as file:
file.write('\nAppended line.')
9. Read a File Line by Line
with open('file.txt', 'r') as file:
for line in file:
print(line.strip())
10. Check if File Exists
import os
exists = os.path.isfile('file.txt')
๐ String Manipulation
11. Reverse a String
reversed_str = my_string[::-1]
12. Check for Palindrome
is_palindrome = my_string == my_string[::-1]
13. Count Occurrences of a Substring
count = my_string.count('substring')
14. Replace Substring
new_string = my_string.replace('old', 'new')
15. Split String into List
words = my_string.split()
๐ Mathematical Operations
16. Calculate Factorial
import math
result = math.factorial(5)
17. Generate Fibonacci Sequence
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
18. Check for Prime Number
def is_prime(n):
return n > 1 and all(n % i for i in range(2, int(n**0.5) + 1))
19. Find GCD of Two Numbers
import math
gcd = math.gcd(a, b)
20. Calculate Square Root
import math
sqrt = math.sqrt(16)
๐ Data Structures
21. Merge Two Dictionaries
merged_dict = {**dict1, **dict2}
22. Invert a Dictionary
inverted = {v: k for k, v in original.items()}
23. Create Dictionary from Two Lists
dict_from_lists = dict(zip(keys, values))
24. Count Frequency of Elements
from collections import Counter
freq = Counter(my_list)
25. Sort Dictionary by Value
sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1]))
๐งช Conditionals and Loops
26. Ternary Conditional Operator
result = 'Yes' if condition else 'No'
27. Enumerate in Loop
for index, value in enumerate(my_list):
print(index, value)
28. Zip Multiple Lists
for a, b in zip(list1, list2):
print(a, b)
29. List Comprehension with Multiple Conditions
result = [x for x in range(100) if x % 2 == 0 and x % 5 == 0]
30. Nested Loops in List Comprehension
pairs = [(x, y) for x in list1 for y in list2]
๐ ๏ธ Utilities
31. Measure Execution Time
import time
start = time.time()
# code block
end = time.time()
print(f"Execution Time: {end - start}")
32. Shuffle a List
import random
random.shuffle(my_list)
33. Generate Random Number
import random
num = random.randint(1, 100)
34. Check if List is Empty
if not my_list:
print("List is empty")
35. Swap Two Variables
a, b = b, a
๐ฆ Modules and Packages
36. Install Package via pip
pip install package_name
37. Import Module with Alias
import numpy as np
38. Handle Import Errors
try:
import module
except ImportError:
# handle error
39. List Installed Packages
pip list
40. Get Current Working Directory
import os
cwd = os.getcwd()
๐งฐ Advanced Techniques
41. Lambda Function
square = lambda x: x * x
42. Map Function
squares = list(map(lambda x: x**2, my_list))
43. Filter Function
evens = list(filter(lambda x: x % 2 == 0, my_list))
44. Reduce Function
from functools import reduce
product = reduce((lambda x, y: x * y), my_list)
45. List All Attributes of an Object
attributes = dir(object)
๐๏ธ Miscellaneous
46. Check Data Type
type_of_var = type(variable)
47. Convert String to Integer
num = int('123')
48. Convert Integer to String
s = str(123)
49. Format String with f-Strings
name = 'Alice'
greeting = f'Hello, {name}!'
50. Use of Any and All
any_true = any([False, True, False])
all_true = all([True, True, True])
These code snippets are practical, often used in day-to-day tasks, and should be part of every Python developer's toolkit. Feel free to bookmark or adapt them to your projects.