12 Tricks To Write Better Python Code
- With Code Example
- April 9, 2024
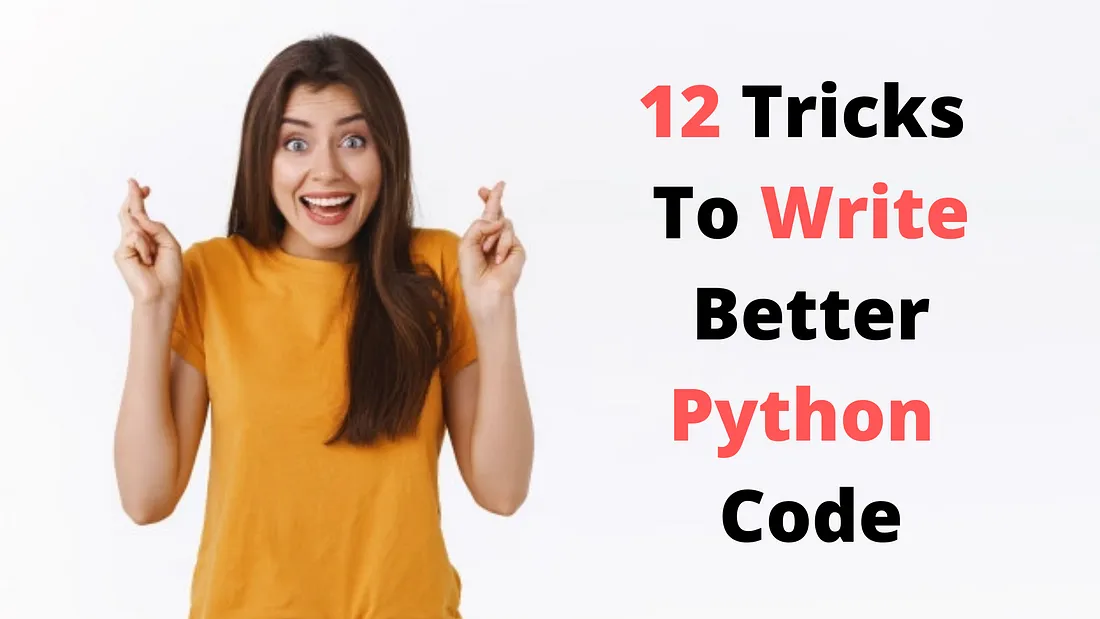
Good day there, This post is a compilation of python coding industry standards that I’ve picked up over the previous several months to help me write more idiomatic Python code.
1. Multiple Assignment
Multiple Assignment is a type of assignment statement in which two or more variables are assigned the same value. In Algol, for example, a:= b:= c:= 0, a, b, and c are all set to zero.
x = 10
y = 10
z = 10
# Instead of this
x = y = z = 10
# Use this
Use this
2. Variable Unpacking
In Python, unpacking is the process of assigning an iterable of values to a tuple (or list) of variables using a single assignment statement.
x, y = [1, 2]
# x = 1, y = 2
x, *y = [1, 2, 3, 4, 5]
# x = 1, y = [2, 3, 4, 5]
x, *y, z = [1, 2, 3, 4, 5]
# x = 1, y = [2, 3, 4], z = 5
3. Swapping Variables
The act of exchanging two variables in computer programming refers to the exchange of the variables’ values. This is usually done using data stored in memory. For example, two variables in a program may be declared as follows (in pseudocode):
swap (x, y); data item x:= 1 data item y:= 0
In python
# Instead of this
temp = x
x = y
y = temp
# Use this
x, y = y, x
4. Name Casing
In python snake_case
is preferred over camelCase
for variables and functions names.
# Instead of this
def isEven(num):
pass# Use this
def is_even(num):
pass
5. Conditional Expressions
The general form of a conditional expression is expr1 is a true-or-false expression. The conditional expression’s value is set to expr2 if the outcome is true. The conditional expression’s value is set to expr3 if the outcome is false.
# Instead of this
def is_even(num):
if num % 2 == 0:
print("Even")
else:
print("Odd")
# Use this
def is_even(num):
print("Even") if num % 2 == 0 else print("Odd")
# Or this
def is_even(num):
print("Even" if num % 2 == 0 else "Odd")
6. String Formatting
String formatting evaluates a string literal containing one or more placeholders using string interpolation (variable substitution), providing a result in which the placeholders are replaced with their corresponding values.
name = "Dumbledore"
item = "Socks"# Instead of this
print("%s likes %s." %(name, item))# Or this
print("{} likes {}.".format(name, item))# Use this
print(f"{name} likes {item}.")
7. Comparison Operator
Operators that compare values and return true or false are known as comparison operators. >, >=, =, ===, and!== are some of the operators… Operators that combine numerous boolean expressions or values into a single boolean output are known as logical operators. &&, ||, and! are examples of operators.
# Instead of this
if 0 < x and x < 1000:
print("x is a 3 digit number")# Use this
if 0 < x < 1000:
print("x is a 3 digit number")
8. Iterating over a list or tuple
We don’t need to use indices to access list elements. Instead, we can do this.
numbers = [1, 2, 3, 4, 5, 6]# Instead of this
for i in range(len(numbers)):
print(numbers[i])# Use this
for number in numbers:
print(number)# Both of these yields the same output
9. Using enumerate()
When you need both indices and values, we can use enumerate()
.
names = ['Harry', 'Ron', 'Hermione', 'Ginny', 'Neville']for index, value in enumerate(names):
print(index, value)
10. Using Set for searching
Searching in a set is faster(O(1)) compared to list(O(n)).
# Instead of this
l = ['a', 'e', 'i', 'o', 'u']def is_vowel(char):
if char in l:
print("Vowel")# Use this
s = {'a', 'e', 'i', 'o', 'u'}def is_vowel(char):
if char in s:
print("Vowel")
11. List comprehension
A list comprehension is a syntactic construct used to create a list from existing lists in several computer languages. In contrast to the use of map and filter functions, it uses the mathematical set-builder notation (set comprehension).
arr = [1, 2, 3, 4, 5, 6]
res = []# Instead of this
for num in arr:
if num % 2 == 0:
res.append(num * 2)
else:
res.append(num)# Use this
res = [(num * 2 if num % 2 == 0 else num) for num in arr]
12. Iterating Dictionary
Using dict.items()
to iterate through a dictionary.
roll_name = {
315: "Dharan",
705: "Priya"
}# Instead of this
for key in roll_name:
print(key, roll_name[key])# Do this
for key, value in roll_name.items():
print(key, value)
Final Words
I have listed top tricks to write python code in a better manner as per the industry standards. I hope these will help you to improve your code quality and if you feel that something is missing or need to add something, feel free to provide your response.