3 Ways To Change Your IP Address With Selenium And Python
- With Code Example
- July 29, 2024
Rotate IP Address with Selenium and Python
Series - Python Selenium
Good day there, Today I’m going to talk about python selenium and how to update your IP address with each visit. This capability is frequently required when testing apps from several IP addresses and regions. I’ll go over a few ways to cycle IP using Python Selenium.
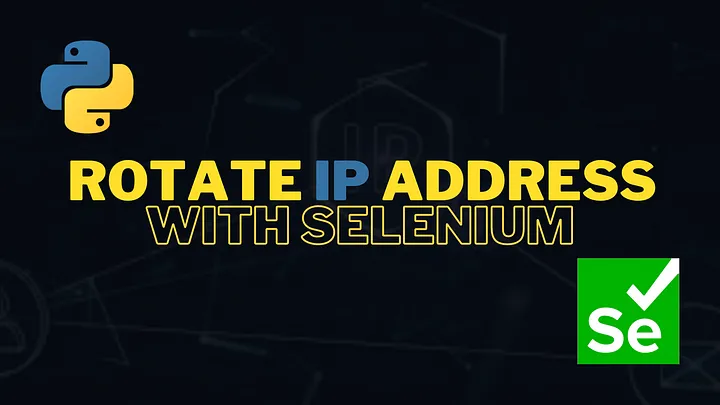
Table of Contents
Using VPN
This is another way to change your Ip by connecting to VPN every time before launching the browser. I am using Windscribe VPN for this example. To start using it we have to install Windscribe in our system.
Signup for Windscribe Account
Click here to signup for a new account with Windscribe.
Add the Windscribe key to apt
Open your terminal by hitting ‘ctrl+alt+t’ and run the following commands:
sudo apt-key adv --keyserver keyserver.ubuntu.com --recv-key FDC247B7
Install Windscribe
echo 'deb [https://repo.windscribe.com/ubuntu](https://repo.windscribe.com/ubuntu) bionic main' | sudo tee /etc/apt/sources.list.d/windscribe-repo.list
sudo apt-get update
sudo apt-get install windscribe-cli
Login to Windscribe
windscribe login
Python script to connect to VPN before launching the Selenium
import os
os.system("windscribe connect") # connect to the random location
os.system("windscribe connect US") # connect to the us location
os.system("windscribe disconnect") # disconnect
Full script
from selenium import webdriver
import osos.system("windscribe connect")chrome = webdriver.Chrome(options=options)
chrome.get("http://ipinfo.io")os.system("windscribe disconnect")
Using free proxy
We can use free available proxies with selenium to change the IP. SSLPROXIES provides a large list of free available proxies. We can use proxies from there in our code below:
from selenium import webdriver
PROXY_STR = "111.222.111.222:1234"
options = webdriver.ChromeOptions()
options.add_argument('--proxy-server=%s' % PROXY_STR)
chrome = webdriver.Chrome(options=options)
chrome.get("http://ipinfo.io")
In the above code, I have defined a variable “PROXY_STR” which contains the Ip and Port. If we need a list of all available proxies for SSLproxies then we have to scrap this website.
from selenium import webdriver
from selenium.webdriver.common.by import By
import chromedriver_autoinstaller # pip install chromedriver-autoinstaller
chromedriver_autoinstaller.install() # To update your chromedriver automatically
driver = webdriver.Chrome()
# Get free proxies for rotating
def get_free_proxies(driver):
driver.get('https://sslproxies.org')
table = driver.find_element(By.TAG_NAME, 'table')
thead = table.find_element(By.TAG_NAME, 'thead').find_elements(By.TAG_NAME, 'th')
tbody = table.find_element(By.TAG_NAME, 'tbody').find_elements(By.TAG_NAME, 'tr')
headers = []
for th in thead:
headers.append(th.text.strip())
proxies = []
for tr in tbody:
proxy_data = {}
tds = tr.find_elements(By.TAG_NAME, 'td')
for i in range(len(headers)):
proxy_data[headers[i]] = tds[i].text.strip()
proxies.append(proxy_data)
return proxies
free_proxies = get_free_proxies(driver)
print(free_proxies)
You’ll get an output in Python dictionary like this:
[{'IP Address': '200.85.169.18',
'Port': '47548',
'Code': 'NI',
'Country': 'Nicaragua',
'Anonymity': 'elite proxy',
'Google': 'no',
'Https': 'yes',
'Last Checked': '8 secs ago'},
{'IP Address': '191.241.226.230',
'Port': '53281',
'Code': 'BR',
'Country': 'Brazil',
'Anonymity': 'elite proxy',
'Google': 'no',
'Https': 'yes',
'Last Checked': '8 secs ago'},
.
.
.
}]
Using chrome extension
This is another way to change your Ip dynamically. I am using the UltraSurf extension which is changing my Ip every time I installed it. So look for the code how it is working.
- Download UltraSurf extension (.crx file).
Click here and copy and paste the following URL — ‘https://chrome.google.com/webstore/detail/ultrasurf-security-privac/mjnbclmflcpookeapghfhapeffmpodij’ and hit the Download button.
- Install extension
from selenium import webdriver
import time
options = webdriver.ChromeOptions()
options.add_extension('path/to/extension.crx')
time.sleep(5)
chrome = webdriver.Chrome(options=options)
chrome.get("http://ipinfo.io")
This will change the IP as the extension is installed.
Conclusion
I’ve given you three options for rotating your IP while using selenium. All of them are free to use, and there are premium plans available if you wish to utilize them without restrictions.
Note: Many websites do not allow automation, as we all know, and I am not advocating for it. This article was prepared only for the sake of education.