Building A React App With Tailwind CSS
- With Code Example
- July 15, 2024
Build Faster, Build Better: Tailwind CSS for React
Beautiful and responsive user interfaces are crucial in the world of modern web development. The way we build web applications has been transformed by React, and it is a juggernaut for creating attractive UIs when paired with Tailwind CSS. This article will discuss how to incorporate Tailwind CSS into your React project and use its utility-first approach to simplify your styling process.
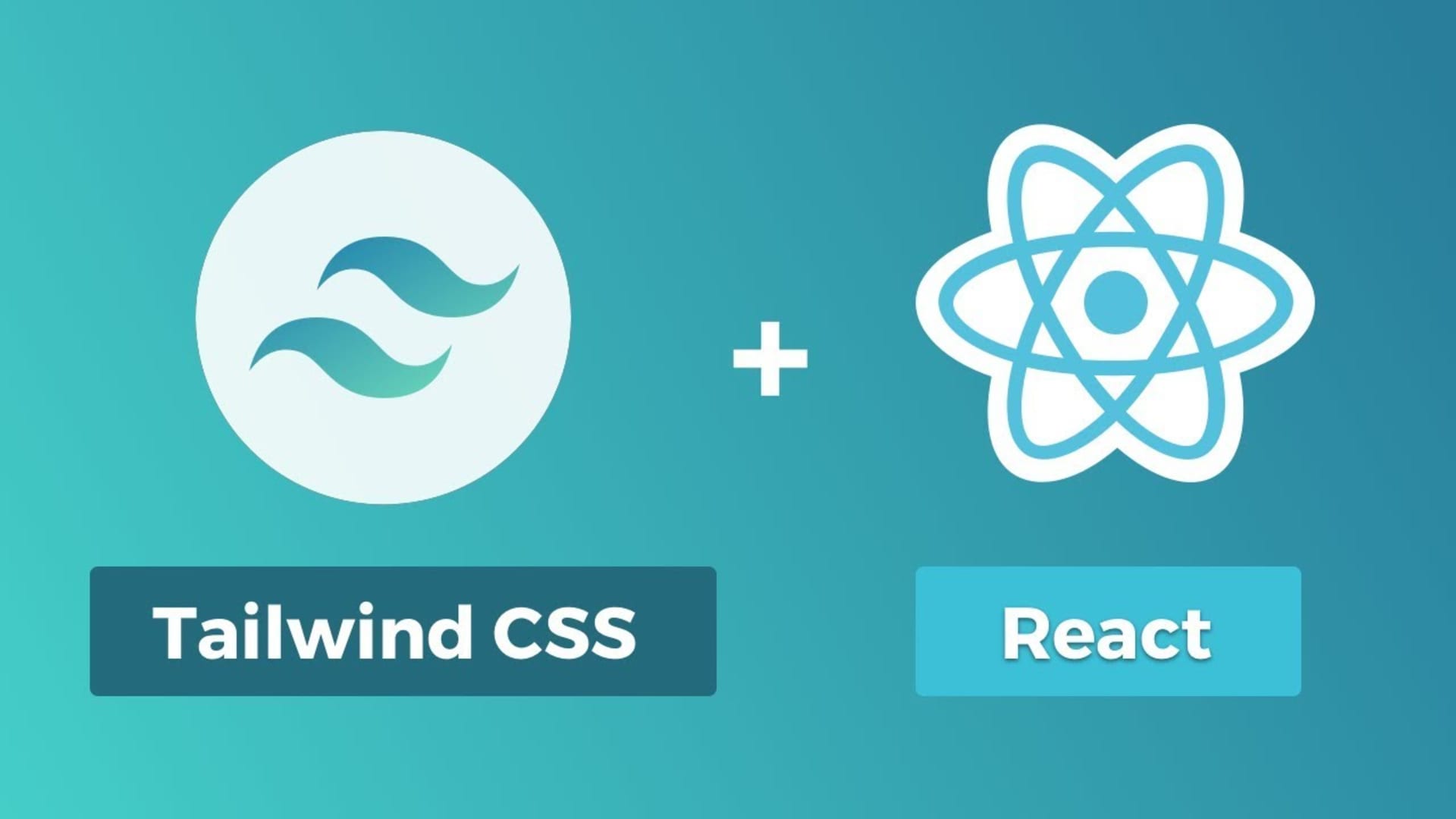
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that provides low-level utility classes to build custom designs without leaving your HTML (or JSX in React’s case). Unlike traditional CSS frameworks that come with predefined components, Tailwind gives you the building blocks to create your own unique designs rapidly.
Why Use Tailwind CSS with React?
- Rapid Development: Tailwind’s utility classes allow you to style elements quickly without writing custom CSS.
- Flexibility: It’s easy to create unique designs without fighting against pre-existing styles.
- Responsive Design: Tailwind makes it simple to create responsive layouts with its intuitive class naming conventions.
- Performance: By purging unused styles in production, Tailwind helps keep your CSS bundle size small.
Setting Up Tailwind CSS in a React Project
Let’s walk through the process of adding Tailwind CSS to a React project:
Step 1: Create a New React Project
If you haven’t already, create a new React project. You can use Create React App or Vite:
# Using Create React App
npx create-react-app my-tailwind-react-app
cd my-tailwind-react-app
# Or using Vite
npm create vite@latest my-tailwind-react-app -- --template react
cd my-tailwind-react-app
npm install
Step 2: Install Tailwind CSS and Its Dependencies
Install Tailwind CSS along with its peer dependencies:
npm install -D tailwindcss postcss autoprefixer
Step 3: Initialize Tailwind CSS
Generate the Tailwind configuration file:
npx tailwindcss init -p
This creates tailwind.config.js
and postcss.config.js
files in your project root.
Step 4: Configure Tailwind
Update the tailwind.config.js
file to include the paths to all of your React components:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Step 5: Add Tailwind Directives to Your CSS
Create or update your main CSS file (often src/index.css
) to include Tailwind’s base, components, and utilities styles:
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 6: Import the CSS File
Make sure your main CSS file is imported in your React application. In src/index.js
or src/main.jsx
, add:
import './index.css';
Using Tailwind CSS in React Components
Now that Tailwind is set up, let’s see how to use it in a React component:
function Button({ children }) {
return (
<button className="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded">
{children}
</button>
);
}
function App() {
return (
<div className="container mx-auto p-4">
<h1 className="text-3xl font-bold underline mb-4">
Hello, Tailwind CSS + React!
</h1>
<Button>Click me</Button>
</div>
);
}
export default App;
In this example, we’ve created a reusable Button
component and styled our App
component using Tailwind utility classes.
Best Practices
Extracting Components: For frequently used patterns, consider extracting them into reusable React components.
Using @apply: For complex classes, use Tailwind’s
@apply
directive in your CSS to create reusable class names:.btn-primary { @apply bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded; }
Responsive Design: Utilize Tailwind’s responsive prefixes (
sm:
,md:
,lg:
,xl:
) to create responsive layouts easily.Dark Mode: Implement dark mode using Tailwind’s dark mode feature by adding
dark:
prefix to classes.
Conclusion
Integrating Tailwind CSS with React provides a powerful combination for building modern, responsive web applications. By leveraging Tailwind’s utility-first approach, you can rapidly prototype and build UIs while maintaining the flexibility to create unique designs.
As you become more comfortable with Tailwind CSS, you’ll find that it not only speeds up your development process but also helps maintain consistency across your project. Remember to explore Tailwind’s extensive documentation to take full advantage of its features and customize it to fit your project’s needs.
Happy coding, and enjoy the synergy of React and Tailwind CSS in your next project!