10 Handy React.js Code Snippets for Your Projects
- With Code Example
- October 3, 2023
Boost Your React.js Development with These Code Snippets
Series - Top In React
Here are 10 useful React.js code snippets that can come in handy for various scenarios. You can adapt and use these snippets in your React projects:
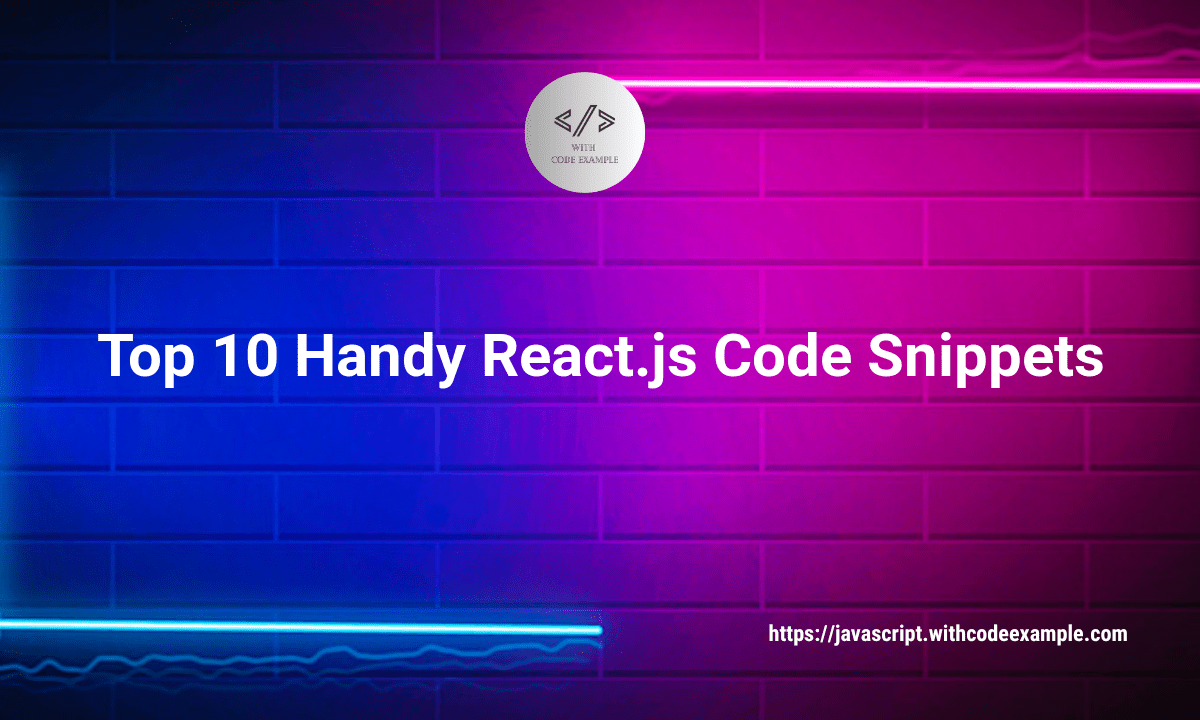
- Creating a Functional Component:
import React from 'react';
function MyComponent() {
return <div>Hello, React!</div>;
}
export default MyComponent;
- Using Props in a Component:
function Greeting(props) {
return <div>Hello, {props.name}!</div>;
}
- Handling State with useState Hook:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
- Mapping Over an Array to Render Components:
const items = ['Item 1', 'Item 2', 'Item 3'];
const ItemList = () => (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
- Conditional Rendering with Ternary Operator:
function Message({ isLoggedIn }) {
return (
<div>
{isLoggedIn ? <p>Welcome back!</p> : <p>Please log in.</p>}
</div>
);
}
- Handling Form Input with State:
import React, { useState } from 'react';
function TextInput() {
const [inputValue, setInputValue] = useState('');
const handleChange = (e) => {
setInputValue(e.target.value);
};
return (
<input
type="text"
value={inputValue}
onChange={handleChange}
placeholder="Enter text"
/>
);
}
- Fetching Data from an API with useEffect:
import React, { useEffect, useState } from 'react';
function DataFetching() {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => setData(data));
}, []);
return <div>{/* Render data here */}</div>;
}
- Using React Router for Routing:
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
</ul>
</nav>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Router>
);
}
- Adding CSS Classes Conditionally:
function Button({ isPrimary }) {
const className = isPrimary ? 'primary-button' : 'secondary-button';
return <button className={className}>Click me</button>;
}
- Handling Click Events:
function handleClick() {
alert('Button clicked!');
}
function ClickButton() {
return <button onClick={handleClick}>Click me</button>;
}
These snippets cover a range of common React.js use cases. Remember to customize them according to your specific project requirements.