JAX Basics: Everything You Need to Know
- With Code Example
- June 4, 2024
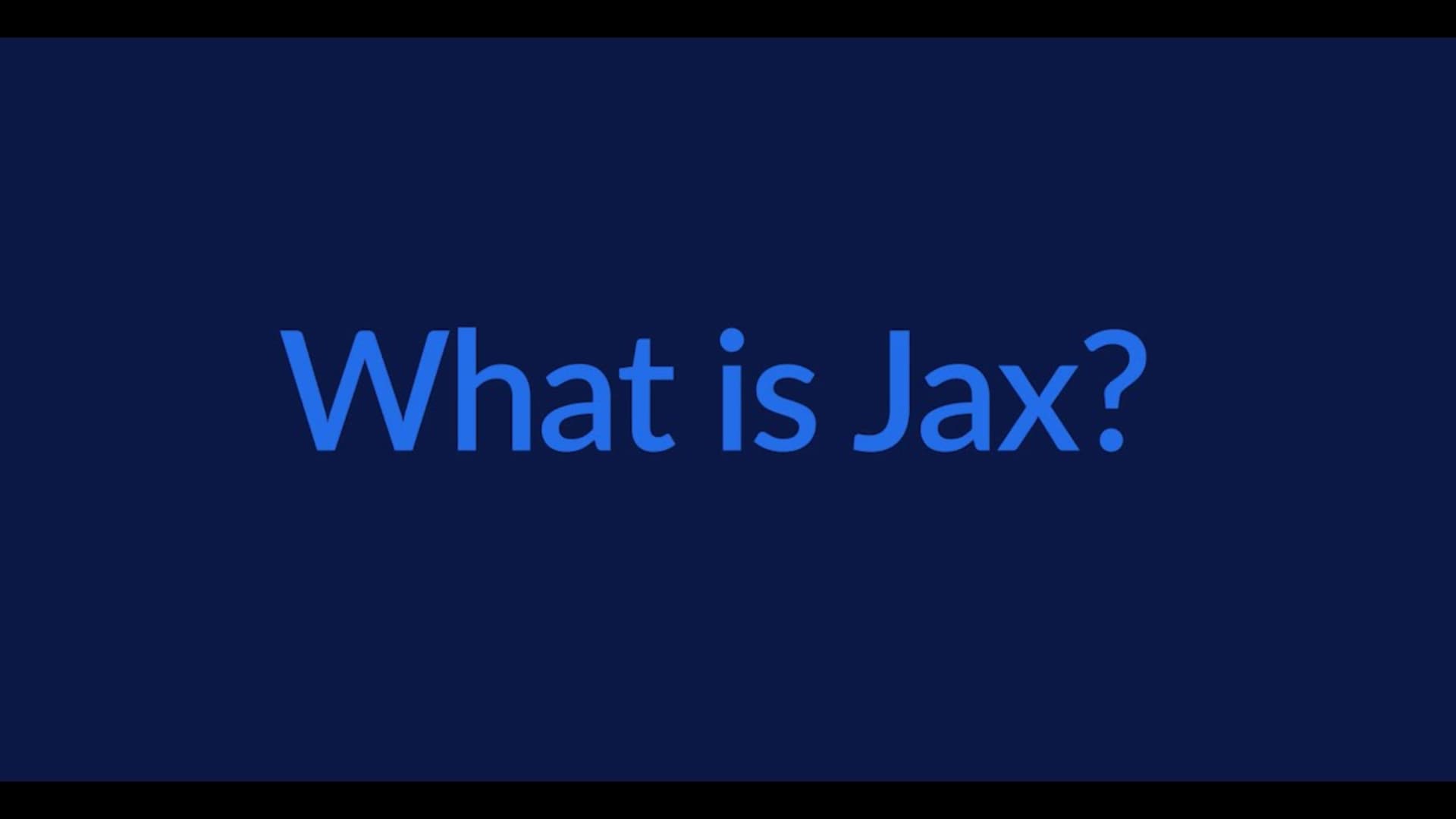
JAX, developed by Google, is a cutting-edge numerical computing framework designed to enhance the efficiency and performance of machine learning (ML) programming. It uniquely integrates two powerful components: Autograd and XLA, providing an innovative solution for automatic differentiation and optimized compilation. This framework is transforming the landscape of ML programming by offering a clean, structured, and intuitive approach to array-based computing.
Table of Contents
Key Components
Autograd:
- Automatic Differentiation: Autograd is a core component of JAX that automates the differentiation process, enabling developers to compute gradients of functions written in Python. This feature is crucial for optimizing ML models, as it simplifies the calculation of derivatives, a common requirement in training algorithms.
- Ease of Use: By leveraging Python’s dynamic capabilities, Autograd allows for seamless integration with existing codebases. This means developers can write their ML models in familiar Python syntax and still benefit from automatic differentiation.
XLA (Accelerated Linear Algebra):
- High-Performance Compilation: XLA compiles high-level operations into low-level, high-performance code suitable for execution on accelerators like GPUs and TPUs. This compilation process significantly enhances the performance of numerical computations by optimizing the execution on hardware accelerators.
- Scalability: XLA’s ability to target various hardware accelerators makes JAX highly scalable, accommodating a range of computational needs from individual researchers to large-scale industrial applications.
Benefits of JAX
- Intuitive API: JAX provides a lightweight API that is highly reminiscent of NumPy, making it accessible to a wide range of users familiar with array-based computing. This similarity ensures that transitioning to JAX requires minimal learning curve for those already accustomed to NumPy’s interface.
- Enhanced Performance: By combining Autograd’s automatic differentiation with XLA’s compilation capabilities, JAX delivers significant performance improvements. This combination allows for efficient computation and rapid prototyping, which are essential in the fast-evolving field of machine learning.
- Flexibility: JAX’s design emphasizes flexibility, allowing developers to experiment with complex models and algorithms. This flexibility is critical for advancing research and development in ML, where novel approaches often require rapid iteration and testing.
Code Examples
Here are some examples to demonstrate how JAX can be used in practice:
- Basic Autograd Example: Computing the gradient of a simple function.
import jax
import jax.numpy as jnp
# Define a simple function
def simple_function(x):
return x**2 + 3*x + 2
# Compute the gradient of the function
grad_function = jax.grad(simple_function)
# Evaluate the gradient at a point
x = 5.0
gradient = grad_function(x)
print(f"The gradient of the function at x={x} is {gradient}")
- Using JAX for Array-Based Operations: Performing operations similar to NumPy.
import jax.numpy as jnp
# Create arrays
a = jnp.array([1.0, 2.0, 3.0])
b = jnp.array([4.0, 5.0, 6.0])
# Perform element-wise addition
c = a + b
print("Array addition result:", c)
# Perform matrix multiplication
matrix_a = jnp.array([[1.0, 2.0], [3.0, 4.0]])
matrix_b = jnp.array([[5.0, 6.0], [7.0, 8.0]])
matrix_c = jnp.dot(matrix_a, matrix_b)
print("Matrix multiplication result:\n", matrix_c)
- Optimizing a Simple Machine Learning Model: Gradient descent optimization.
import jax
import jax.numpy as jnp
from jax import grad
# Define a simple quadratic loss function
def loss(w, x, y):
return jnp.sum((w * x - y) ** 2)
# Generate some sample data
x_data = jnp.array([1.0, 2.0, 3.0, 4.0])
y_data = jnp.array([2.0, 4.0, 6.0, 8.0])
# Initialize weights
w = jnp.array(0.0)
# Compute the gradient of the loss function with respect to w
grad_loss = grad(loss)
# Perform gradient descent
learning_rate = 0.01
for i in range(100):
w_grad = grad_loss(w, x_data, y_data)
w -= learning_rate * w_grad
print(f"Optimized weight: {w}")
Conclusion
JAX represents a significant advancement in the field of numerical computing and machine learning, merging the strengths of automatic differentiation and high-performance compilation. Developed by Google, this framework not only enhances performance but also provides an intuitive and flexible platform for researchers, developers, and educators. As machine learning continues to grow in importance across various industries, tools like JAX will be instrumental in driving innovation and efficiency.
By simplifying complex processes and offering robust scalability, JAX is poised to become a cornerstone in the toolkit of modern ML practitioners, ensuring that high-performance numerical computing is more accessible and effective than ever before.