JSON, The Data Format Powering Our Digital World
- With Code Example
- June 22, 2024
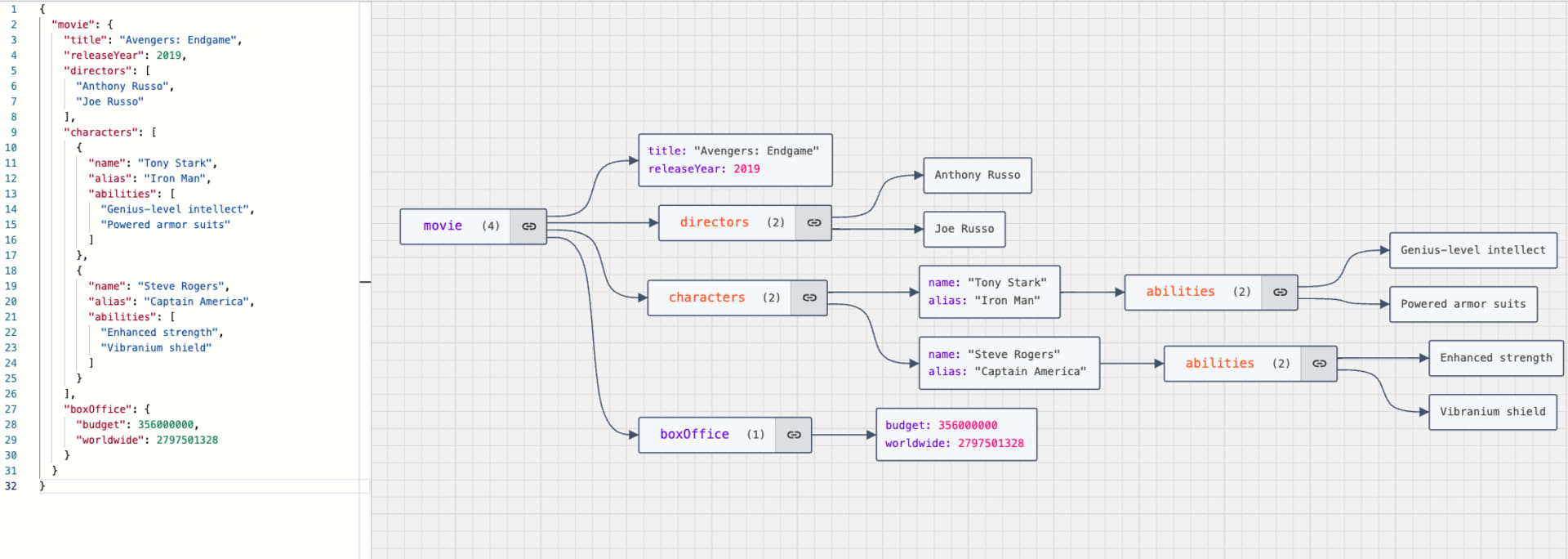
In the vast landscape of web technologies, JSON stands out as a quiet yet indispensable player. But what exactly is JSON, and why has it become so crucial in modern web development? Let’s embark on a journey to uncover the secrets of this ubiquitous data format.
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data interchange format that’s easy for humans to read and write and easy for machines to parse and generate. Despite its name, JSON is language-independent and can be used with most modern programming languages.
Born from the need for a real-time server-to-browser communication protocol, JSON has evolved into a go-to solution for storing and transporting data across the web. Its simplicity and flexibility have made it a favorite among developers worldwide.
The Building Blocks of JSON
At its core, JSON uses two primary structures:
- Objects: Unordered collections of key-value pairs
- Arrays: Ordered lists of values
Let’s break these down with some examples:
1. JSON Objects:
{
"name": "Tony Stark",
"age": 45,
"isAvenger": true,
"suits": ["Mark I", "Mark II", "Mark III"],
"company": {
"name": "Stark Industries",
"founded": 1940
}
}
This JSON object represents Tony Stark. Notice how it contains various data types:
- Strings: “Tony Stark”, “Mark I”, etc.
- Numbers: 45, 1940
- Booleans: true
- Arrays: [“Mark I”, “Mark II”, “Mark III”]
- Nested objects: The “company” object
2. JSON Arrays:
[
{
"name": "Steve Rogers",
"alias": "Captain America"
},
{
"name": "Natasha Romanoff",
"alias": "Black Widow"
},
{
"name": "Bruce Banner",
"alias": "Hulk"
}
]
This JSON array contains a list of Avengers, each represented by an object.
JSON Syntax Rules:
- Data is in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
- Strings must be in double quotes
The Power of Nesting
One of JSON’s strengths is its ability to represent complex data structures through nesting. Let’s look at a more intricate example:
{
"movie": {
"title": "Avengers: Endgame",
"releaseYear": 2019,
"directors": ["Anthony Russo", "Joe Russo"],
"characters": [
{
"name": "Tony Stark",
"alias": "Iron Man",
"abilities": ["Genius-level intellect", "Powered armor suits"]
},
{
"name": "Steve Rogers",
"alias": "Captain America",
"abilities": ["Enhanced strength", "Vibranium shield"]
}
],
"boxOffice": {
"budget": 356000000,
"worldwide": 2797501328
}
}
}
This nested structure allows us to represent complex relationships and hierarchies within our data, making JSON incredibly versatile for a wide range of applications.
Why JSON Dominates the Web
Lightweight and Fast: JSON’s minimalist syntax means less data to transfer, resulting in faster transmission times.
Human-Readable: Unlike some data formats, JSON is easy for humans to read and write, making debugging and manual data entry much simpler.
Language Independence: While it originated from JavaScript, JSON can be used with virtually any modern programming language.
Flexibility: JSON can represent simple key-value pairs or complex nested structures, adapting to various data needs.
Wide Support: Most major browsers and web technologies have built-in support for JSON parsing and generation.
Real-World Applications of JSON
API Communication: JSON is the de facto standard for API responses. When you fetch data from Twitter, Facebook, or virtually any modern web service, you’re likely receiving JSON.
Configuration Files: Many applications use JSON for configuration files due to its readability and ease of parsing.
NoSQL Databases: Some NoSQL databases, like MongoDB, store data in a JSON-like format.
Data Storage: JSON is often used to store structured data, from user preferences to application states.
Cross-Origin Resource Sharing (CORS): JSON with Padding (JSONP) is a technique used to request data from a server in a different domain, circumventing same-origin policy restrictions.
JSON vs. XML: A Brief Comparison
Before JSON, XML (eXtensible Markup Language) was the go-to format for data interchange. While XML is still used in many applications, JSON has several advantages:
- Simplicity: JSON has a simpler syntax and is generally more concise than XML.
- Parsing Speed: JSON is typically faster to parse than XML, especially in browsers.
- Data Types: JSON supports data types like numbers and booleans natively, while XML treats everything as strings.
Here’s a quick comparison:
JSON:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
XML:
<person>
<name>John Doe</name>
<age>30</age>
<city>New York</city>
</person>
As you can see, the JSON version is more compact and arguably easier to read.
Working with JSON in Different Languages
While JSON originated from JavaScript, it’s supported by most modern programming languages. Here are a few examples:
JavaScript:
// Parsing JSON
const data = JSON.parse('{"name": "John", "age": 30}');
console.log(data.name); // Output: John
// Stringifying JSON
const obj = {name: "John", age: 30};
const json = JSON.stringify(obj);
console.log(json); // Output: {"name":"John","age":30}
Python:
import json
# Parsing JSON
data = json.loads('{"name": "John", "age": 30}')
print(data['name']) # Output: John
# Stringifying JSON
obj = {'name': 'John', 'age': 30}
json_string = json.dumps(obj)
print(json_string) # Output: {"name": "John", "age": 30}
Java:
import org.json.JSONObject;
// Parsing JSON
JSONObject obj = new JSONObject("{\"name\":\"John\",\"age\":30}");
String name = obj.getString("name");
System.out.println(name); // Output: John
// Creating JSON
JSONObject newObj = new JSONObject();
newObj.put("name", "John");
newObj.put("age", 30);
System.out.println(newObj.toString()); // Output: {"name":"John","age":30}
JSON Security Considerations
While JSON is incredibly useful, it’s important to be aware of potential security issues:
JSON Injection: Similar to SQL injection, malicious JSON payloads can potentially exploit vulnerabilities in poorly designed systems.
Sensitive Data: Be cautious about storing sensitive information in JSON, especially if it’s being transmitted or stored insecurely.
Parsing Vulnerabilities: Some JSON parsers have had vulnerabilities that could lead to denial-of-service attacks or remote code execution.
Conclusion
JSON’s simplicity, flexibility, and widespread support have made it an integral part of the modern web ecosystem. From powering API communications to storing configuration data, JSON’s impact on how we structure and transmit information cannot be overstated.
As you continue your journey in web development or data engineering, you’ll undoubtedly encounter JSON at every turn. Embrace its elegance, understand its nuances, and you’ll have a powerful tool at your disposal for handling data in the digital age.
Remember, behind every sleek web application or mobile app you use, there’s likely some JSON working tirelessly behind the scenes, ensuring that data flows smoothly across the digital landscape.