Top Javascript Coding Round Questions For Beginners - 2
- With Code Example
- August 7, 2024
Balanced Parentheses, the sum of All Numbers, the Longest Word, and the Fibonacci Sequence
Series - Javascript Interview
This post is a continuation of my previous post for “Top Javascript Coding Round Questions For Beginners”. This is part 2 of this series and will talk about some more important javascript interview questions for the coding round and provide the working code example and explanation. Through these posts, my main agenda is to teach the beginners for the coding rounds and make them job-ready. So without wasting the time, let’s get started…
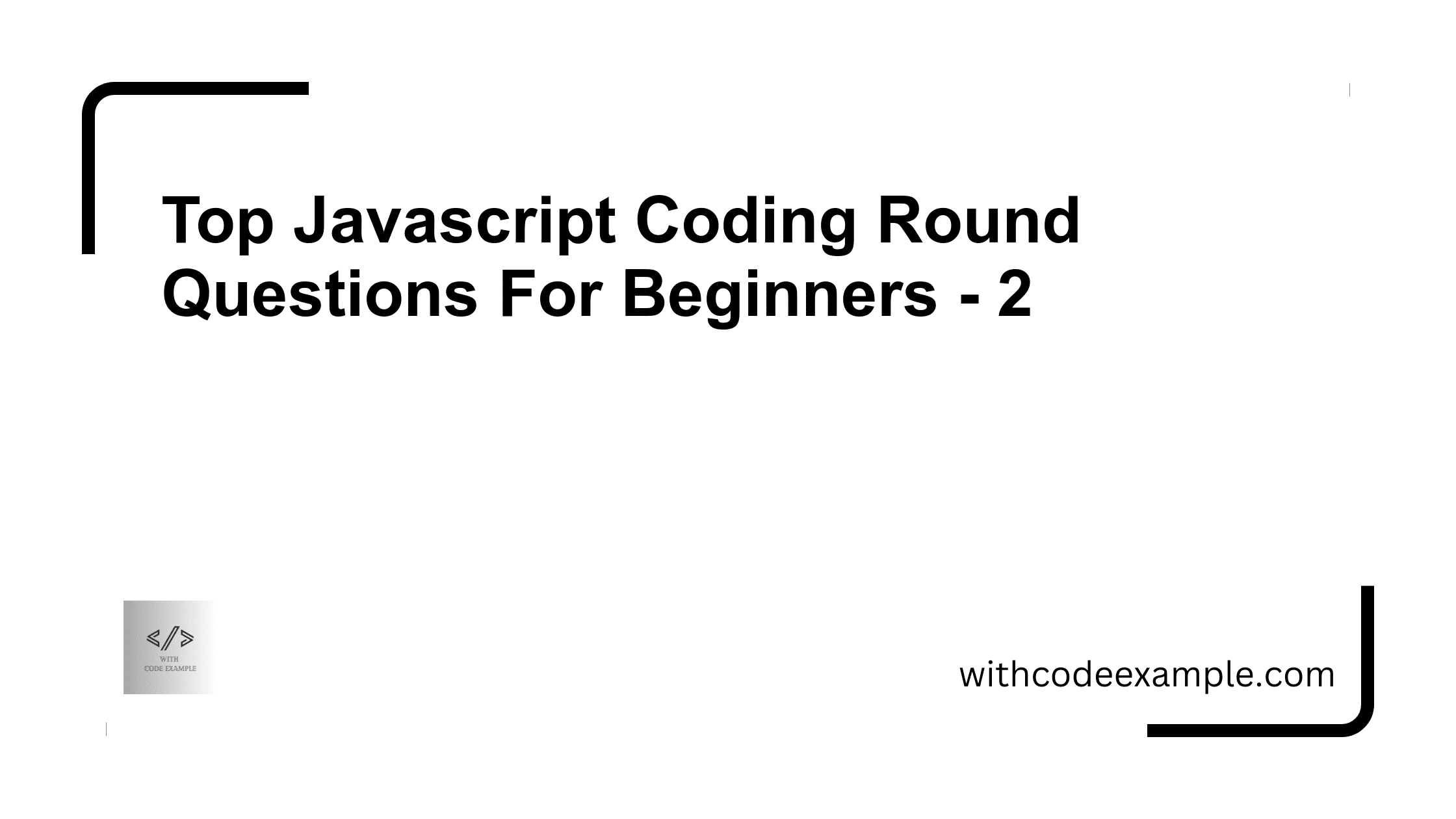
Table of Contents
5. Check for Balanced Parentheses
Write a function that takes a string input of Parentheses and checks if they are closed properly or not. That means every open bracket should have a closing bracket along with it. This is a very common thing while coding we have to check it manually, let’s write a code for it.
function isValid(s) {
const stack = [];
const map = {
'(': ')',
'[': ']',
'{': '}'
};
for (let char of s) {
if (map[char]) {
stack.push(char);
} else {
const last = stack.pop();
if (map[last] !== char) {
return false;
}
}
}
return stack.length === 0;
}
Explanation
In the code, there is a function called isValid
which takes taking string as input and returns the boolean according to the Parentheses balanced. It uses a pattern stored in the map, with the string over each iteration of the string and if it is in the correct order then it will push in the stack otherwise remove it from the stack.
6. The sum of All Numbers in a Range
Write a javascript function that takes two numbers as input and returns the sum of all numbers between them. For example, if I input 2 and 5, then it will return the sum of 2+3+4+5 = 14. See the code below…
function sumAll(arr) {
const [min, max] = arr.sort((a, b) => a - b);
let sum = 0;
for (let i = min; i <= max; i++) {
sum += i;
}
return sum;
}
// Example usage:
sumAll([1, 4]); // 10 (1 + 2 + 3 + 4)
Explanation
In this piece of code, we are taking an array as input in sumAll
function and returning the sum of all array numbers to do that, we are iterating over the range of elements by sorting them in ascending order, and each iteration increases the value of the total sum. Finally, it will return the total after the for-loop exit.
7. Find the Longest Word in a String
So this task is to write a function that will return the longest word in the given string. It is straightforward to understand the problem, with no fancy words used. For example, if we input “Hello friends, How are you?”, then it will return “friends” because “friends” is the longest word in this sentence.
function findLongestWord(str) {
const words = str.split(' ');
let longestWord = '';
for (let word of words) {
if (word.length > longestWord.length) {
longestWord = word;
}
}
return longestWord;
}
Explanation
The following code takes a string as input in findLongestWord
function and returns the longest string from the string. To do it it splits the string into an array of words and then it checks for the length of each word in the array with a loop if it is greater than the previous word it replaces it with new and moves to the next iteration.
8. Fibonacci Sequence
In mathematics, the Fibonacci sequence is a sequence in which each number is the sum of the two preceding ones. Numbers that are part of the Fibonacci sequence are known as Fibonacci numbers, commonly denoted Fn. The sequence commonly starts from 0 and 1, although some authors start the sequence from 1 and 1 or sometimes (as did Fibonacci) from 1 and 2. Starting from 0 and 1, the sequence begins. wikipedia
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, ….
// Approach 1
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Approach 2
// For better performance, an iterative approach can be used:
function fibonacciIterative(n) {
let a = 0, b = 1, temp;
for (let i = 2; i <= n; i++) {
temp = a + b;
a = b;
b = temp;
}
return n ? b : a;
}
Explanation
Approach 1:
The nth Fibonacci number can be found using the recursive `fibonacci} function. It uses a base case to return the value for ( n ) if ( n ) is 0 or 1. It iteratively breaks down the issue until it reaches the basic case, returning the sum of the Fibonacci numbers at places (n-1 ) and (n-2 ) for values of (n ) higher than 1.
Approach 2:
Using an iterative method, the fibonacciIterative
function determines the nth Fibonacci number. In order to represent the first two Fibonacci numbers, 0 and 1, it initializes two variables, {a} and {b}. Next, iterating from 2 to {n}, it updates these variables each time to reflect the subsequent Fibonacci number. Before updating {a} to {b} and {b} to {temp}, the sum of {a} and {b} is momentarily stored in the variable {temp}. Lastly, if {n} is not zero, it returns {b}; if not, it returns {a}.