Building a Robust Gin Example Project: A Guide for Go Developers
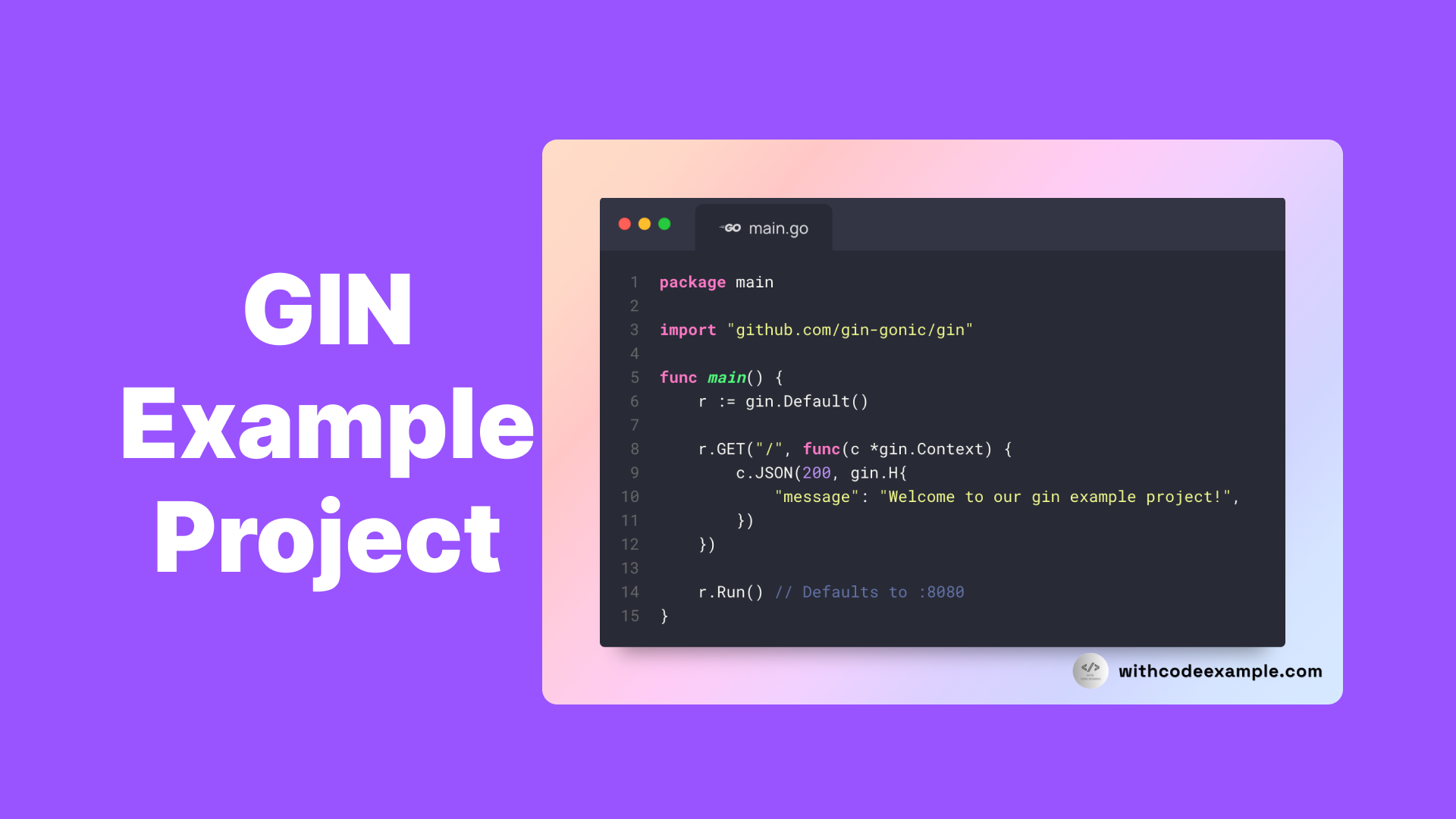
If you’re diving into Go (Golang) web development, the Gin framework is a powerful tool to build high-performance APIs and web applications. In this guide, we’ll create a gin example project from scratch, covering everything from setup to advanced features. Whether you’re a beginner or looking to refine your skills, this tutorial will help you harness Gin’s speed and simplicity.
Why Choose Gin for Your Go Projects?
Gin is a lightweight yet feature-rich framework that excels in speed and efficiency. It’s built on top of Go’s net/http
package and includes essential tools like routing, middleware support, and error handling. By the end of this gin example project, you’ll understand how to:
- Set up a Gin server
- Create RESTful APIs
- Use middleware for logging, authentication, and error handling
- Structure your project for scalability
Prerequisites
Before starting your gin example project, ensure you have:
- Go installed (version 1.16 or higher)
- Basic knowledge of Go syntax
- A code editor (VS Code, GoLand, etc.)
Step 1: Project Setup
Let’s initialize a new Go module and install Gin.
- Create a project directory:
```bash
mkdir gin-example-project
cd gin-example-project
2. **Initialize the Go module**:
bash
go mod init github.com/your-username/gin-example-project
3. **Install Gin**:
bash
go get -u github.com/gin-gonic/gin
## Step 2: Building a Basic Gin Server
Start with a simple "Hello, World!" server.
Create a `main.go` file:
go
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "Welcome to our gin example project!",
})
})
r.Run() // Defaults to :8080
}
Run the server:
bash
go run main.go
Visit `http://localhost:8080` to see the JSON response.
## Step 3: Adding Routes and Controllers
Expand your **gin example project** by creating RESTful endpoints. Let’s build a task management API.
### Define a Task Struct
Add this to `main.go`:
go
type Task struct {
ID string json:"id"
Title string json:"title"
Done bool json:"done"
}
var tasks = []Task{
{ID: "1", Title: "Learn Go", Done: false},
{ID: "2", Title: "Build a Gin Project", Done: false},
}
### Create Task Routes
go
func main() {
r := gin.Default()
// Existing route
r.GET("/", func(c *gin.Context) { ... })
// New routes
r.GET("/tasks", getTasks)
r.POST("/tasks", createTask)
r.GET("/tasks/:id", getTaskByID)
r.Run()
}
// Handler functions
func getTasks(c *gin.Context) {
c.JSON(200, tasks)
}
func createTask(c *gin.Context) {
var newTask Task
if err := c.ShouldBindJSON(&newTask); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
tasks = append(tasks, newTask)
c.JSON(201, newTask)
}
func getTaskByID(c *gin.Context) {
id := c.Param("id")
for _, task := range tasks {
if task.ID == id {
c.JSON(200, task)
return
}
}
c.JSON(404, gin.H{"error": "Task not found"})
}
Test the endpoints using tools like **Postman** or **curl**.
## Step 4: Integrating Middleware
Gin’s middleware system lets you add functionality like logging, authentication, or rate limiting.
### Example: Logging and Recovery
Gin’s default middleware already includes logging and recovery. To add custom middleware:
go
func LoggerMiddleware() gin.HandlerFunc {
return func(c *gin.Context) {
fmt.Printf("Request: %s %s\n", c.Request.Method, c.Request.URL.Path)
c.Next()
}
}
func main() {
r := gin.New()
r.Use(LoggerMiddleware(), gin.Recovery()) // Override default middleware
// ... routes
}
## Step 5: Structuring Your Gin Example Project
For scalability, organize your code into separate packages:
/gin-example-project
├── handlers/
│ └── tasks.go
├── models/
│ └── task.go
├── main.go
├── go.mod
└── go.sum
1. **models/task.go**: Define the `Task` struct and database logic.
2. **handlers/tasks.go**: Move route handlers here.
## Step 6: Adding Database Integration
For a real-world **gin example project**, connect to a database like PostgreSQL.
1. Install a driver:
bash
go get -u github.com/lib/pq
```
- Update
models/task.go
to include database operations.
Tips for Enhancing Your Gin Project
- Validation: Use Gin’s built-in validation or
go-playground/validator
. - Testing: Write unit tests with
net/http/httptest
. - Deployment: Containerize with Docker or deploy to platforms like Heroku.
Conclusion
In this gin example project, you’ve built a RESTful API with routing, middleware, and scalable structuring. Gin’s simplicity and performance make it ideal for everything from microservices to full-stack apps. Use this foundation to explore advanced features like WebSocket support or gRPC integration.
Ready to share your own gin example project? Dive deeper by adding authentication, frontend integration, or caching. The possibilities are endless with Go and Gin!