Building Scalable Microservices with Go and Kubernetes
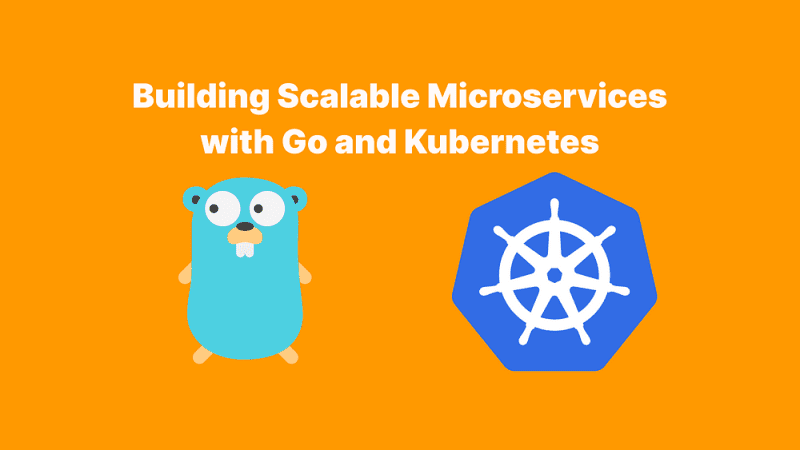
SummaryLearn how to build scalable microservices using Go and Kubernetes. Discover the benefits of microservices architecture and why Go and Kubernetes are ideal choices. This guide covers building a microservice with Go, deploying to Kubernetes, and scaling and managing microservices. You will also learn best practices and considerations for mastering microservice-based architecture, including breaking down complex systems and unlocking the secrets of microservice architecture.
Hello Developers, in today’s post, we will build a scalable microservice using Golang and Kubernetes. We will discuss the benefits of using Go for microservices, an introduction to Kubernetes and its features, and will show by step-by-step guide on how to build, containerize, and deploy the application.
Building Scalable Microservices with Go and Kubernetes
Microservices architecture
Microservices architecture has gained a lot of popularity in recent times, especially for building large-scale systems like Amazon, Netflix, Uber, and Spotify. In microservice architecture, we develop each service to perform a specific task, and it can be scaled, developed, and deployed independently. This approach is beneficial for flexibility, resilience, and scalability.
# Unlock The Secrets Of Microservice Architecture
Unlock The Secrets Of Microservice Architecture
Breaking Down Complex Systems: A Simple Guide to Mastering MicroService-Based Architecture
To build a scalable microservice, choosing the right programming language is very crucial. Go (also known as Golang) has become the popular choice to develop microservice development. Golang has a lot of unique qualities, including simplicity, performance, and concurrency.
10 Go Language Features Every Developer Should Know
Kubernetes has also become the most popular orchestration tool to run these microservices. Kubernetes provides a powerful platform to automate deployment, scale, and manage microservices.
Why Go for Microservices?
Golang provides tons of features that make it outstanding and make it the best choice to develop microservices with it; some of them are listed below -
- Concurrency: Go provides concurrency by default, which allows us to write programs that can perform multiple tasks simultaneously. This is very helpful for microservices because each microservice can handle multiple requests concurrently.
- Simplicity: Go use simple and clean syntax, which makes it easy to learn, read, write, and maintain code. Its simplicity makes it the first choice for developers to work with.
- Performance: Golang is a statically typed programming language that compiles to machine code, which allows golang to provide excellent performance, which is most important for microservices.
- Lightweight: Golan’s binaries are statically linked, which means that they don’t need any external dependencies to run. This makes it easy to containerize and deploy.
Why Kubernetes for Orchestration?
Kubernetes has become the best choice for container orchestration in recent days, which provides a powerful platform to automate your deployment, scaling, and management of containers. Here are some features of Kubernetes that stand out for microservices —
- Scalability: Kubernetes provides the auto-scaling feature based on CPU and Memory use. Which makes the service run without any interruption.
- High Availability: It provides features like self-healing and rolling updates to ensure the high availability of your services.
- Automation: Kubernetes provide the automation platform to deploy scale and manage the microservices.
Building a Microservice with Go
Let’s create a simple Go microservice Resuful api using the golang to manage the bookstore —
Create a new Go application and create a new file inside this application
go mod init kubernetes-tutorial
main.go
package main
import (
"encoding/json"
"log"
"net/http"
"github.com/gorilla/mux"
)
type Book struct {
ID string `json:"id"`
Title string `json:"title"`
Author string `json:"author"`
}
var books []Book
func main() {
// Initialize the router
router := mux.NewRouter()
// Define the books slice
books = append(books, Book{ID: "1", Title: "Book One", Author: "John Doe"})
books = append(books, Book{ID: "2", Title: "Book Two", Author: "Jane Doe"})
// Define the API endpoints
router.HandleFunc("/books", getBooks).Methods("GET")
router.HandleFunc("/books/{id}", getBook).Methods("GET")
// Start the server
log.Fatal(http.ListenAndServe(":8000", router))
}
func getBooks(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(books)
}
func getBook(w http.ResponseWriter, r *http.Request) {
params := mux.Vars(r)
for _, book := range books {
if book.ID == params["id"] {
json.NewEncoder(w).Encode(book)
return
}
}
json.NewEncoder(w).Encode(&Book{})
}
This sample code will create the restful api, which will listen to the port:8000. You can run it using go run main.go
.
Now we need to Dockerize the application using the Dockerfile
.Dockerfile
# Use the official Go image as the base
FROM golang:alpine
# Set the working directory
WORKDIR /app
# Copy the Go code
COPY . .
# Build the Go binary
RUN go build -o main main.go
# Expose the port
EXPOSE 8000
# Run the command to start the service
CMD ["./main"]
To build the Docker image, we have to run the following command:
docker build -t books-service .
This command will build the Docker image with the tag books-service
.
Deploying to Kubernetes
To deploy the containerized microservice to a Kubernetes cluster, we will need to create a deployment file that defines the configuration of the deployment.
Here is the example YAML file:
deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: books-service
spec:
replicas: 5
selector:
matchLabels:
app: books-service
template:
metadata:
labels:
app: books-service
spec:
containers:
- name: books-service
image: books-service:latest
ports:
- containerPort: 8000
In the following file, we have defined books-service with 5 replicas using the books-service:latest docker image.
To apply the deployment, run the following command:
kubectl apply -f deployment.yaml
This Kubernetes command will create the deployment and its resources in the Kubernetes cluster.
Scaling and Managing Microservices with Kubernetes
To scale the applications, Kubernetes provides many features like —
- HPA (Horizontal Pod Autoscaling): It will automatically increase and decrease the replicas based on the CPU, Memory or Other metrics.
- Rolling Updates: This feature provides the power to rollout the updates without any downtime to ensure the high availability of the microservice.
To scale the deployment, we have to run the following command:
kubectl scale deployment books-service --replicas=10
This command will increase the replicas to 10.
If you want to update the image of the deployment then you have run the following command —
kubectl rollout update deployment books-service --image=books-service:latest
Best Practices and Considerations
To build highly scalable and robust microservices with Go and Kubernetes, we have to follow the following best practices:
- Service Discovery: Implement the service discovery the establish the communication between the microservices
- Monitoring and Logging: Implement the proper logging and monitoring to troubleshoot issues and measure performance.
- Security: Use security measures to protect your microservices from unauthorized access and data leaks.
- Networking: Implement networking policies to control the traffic flow between microservices.
Conclusion
In this blog post, we have explored building microservices using Go and Kubernetes. We also discussed the benefits of using Go and Kubernetes, which will help you choose the right tools for your next project. I have also provided real real-world example to create your first microservice and deploy it.
Thank you for taking the time to read this article! If you found it helpful, a couple of claps 👏 👏 👏 would be greatly appreciated — it motivates me to continue writing more. If you want to learn more about open-source and full-stack development, follow me on Twitter (X) and Medium.