Confluent Kafka Go: Building Real-Time Streaming Apps with Golang
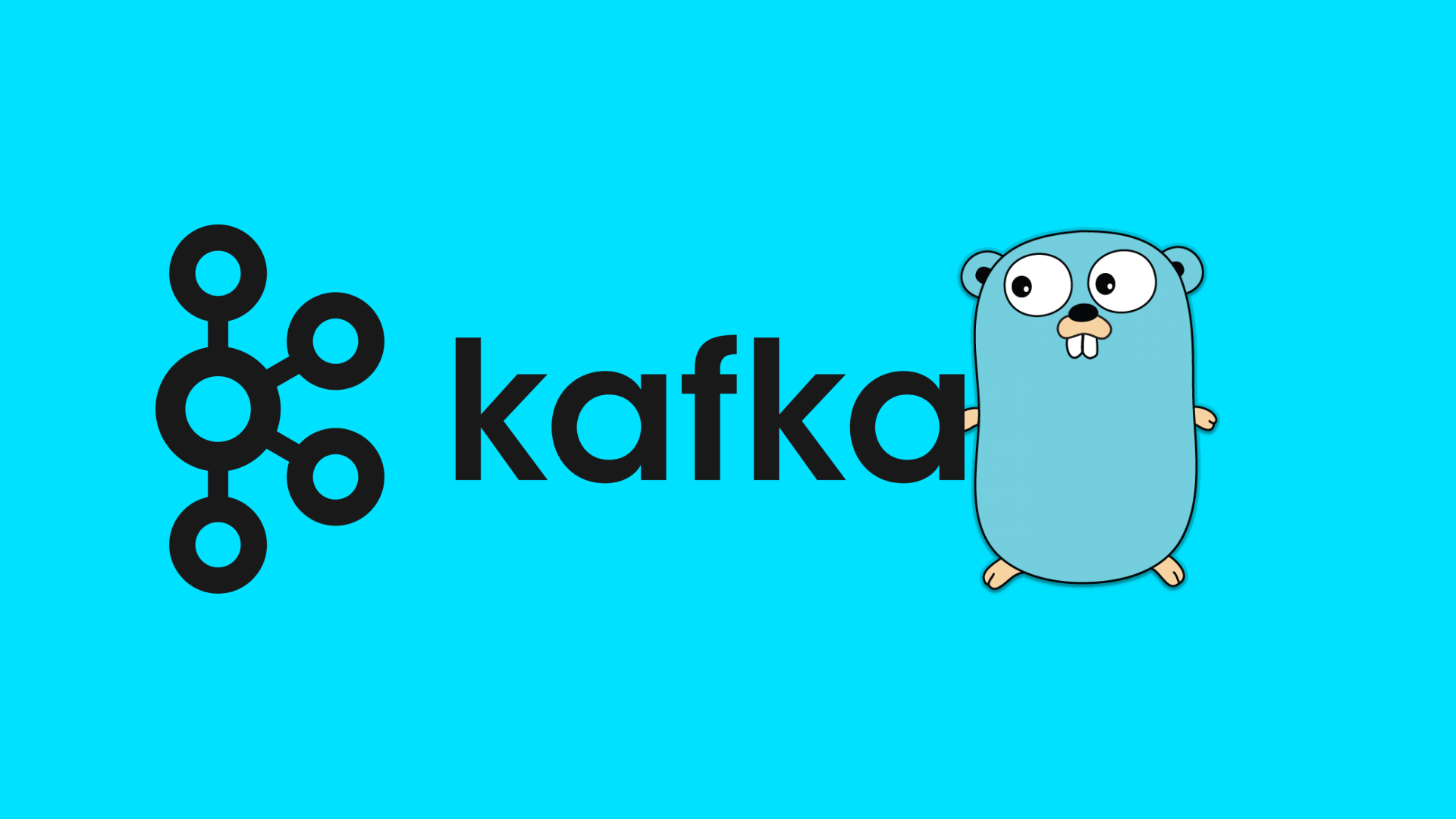
What Is Confluent Kafka Go?
Confluent Kafka Go is the official Golang client library for Apache Kafka®, developed and maintained by Confluent. It provides a robust and efficient way for Go developers to interact with Kafka, enabling seamless integration with the broader Confluent ecosystem, including Kafka Connect, Kafka Streams, and Schema Registry.
With Confluent Kafka Go, you can:
- Produce and consume messages efficiently, leveraging Go's powerful concurrency model.
- Work with schemas (Avro, JSON, Protobuf) through Confluent Schema Registry, ensuring data consistency.
- Manage Kafka clusters effortlessly, whether on Confluent Cloud or a self-hosted environment.
- Leverage advanced features like Exactly-Once Semantics (EOS) and transactional messaging for reliable event-driven architectures.
Why Use Confluent Kafka Go?
- High Performance: Optimized for low-latency and high-throughput message processing.
- Seamless Confluent Ecosystem Integration: Works effortlessly with Schema Registry, REST Proxy, and ksqlDB.
- Strong Type Safety: Ensures structured data handling via serializers/deserializers (SerDes).
- Cloud-Native Capabilities: Supports security, monitoring, and scalability on Confluent Cloud.
- Reliability & Fault Tolerance: Features like idempotent producers and transactional messaging ensure message consistency.
Getting Started with Confluent Kafka Go
1. Install the Library
Use the Confluent-maintained confluent-kafka-go
package:
go get github.com/confluentinc/confluent-kafka-go/kafka
2. Configure a Kafka Producer
Set up a producer to send messages to Kafka:
producer, err := kafka.NewProducer(&kafka.ConfigMap{
"bootstrap.servers": "pkc-12345.confluent.cloud:9092",
"security.protocol": "SASL_SSL",
"sasl.mechanism": "PLAIN",
"sasl.username": "API_KEY",
"sasl.password": "API_SECRET",
})
3. Configure a Kafka Consumer
Set up a consumer to read messages from Kafka:
consumer, err := kafka.NewConsumer(&kafka.ConfigMap{
"bootstrap.servers": "localhost:9092",
"group.id": "my-group",
"auto.offset.reset": "earliest",
})
Advanced Confluent Kafka Go Features
Schema Registry Integration
Serialize and deserialize Avro messages using github.com/confluentinc/confluent-kafka-go/schemaregistry
:
srClient := schemaregistry.NewClient(schemaregistry.NewConfig("https://sr-endpoint.confluent.cloud"))
serializer := NewAvroSerializer(srClient, 5) // Using schema ID 5
Exactly-Once Semantics (EOS)
Ensure message processing guarantees with transactional writes:
producer, _ := kafka.NewProducer(&kafka.ConfigMap{
"transactional.id": "my-tx-id",
})
producer.InitTransactions()
producer.BeginTransaction()
Monitoring & Logging
Use Confluent Control Center or external monitoring tools like Prometheus and Grafana for visibility into Kafka performance.
Best Practices for Confluent Kafka Go
1. Connection Management
Efficient connection management is key to maintaining a stable and performant Kafka setup. Creating a new producer or consumer for every operation is inefficient and can lead to resource exhaustion. Instead:
- Reuse Producers and Consumers: Initialize Kafka producers and consumers once and reuse them throughout the application lifecycle.
- Implement Connection Pooling: If handling multiple requests, use a connection pool to manage producer and consumer instances efficiently.
- Graceful Shutdown: Ensure proper cleanup of connections by closing producers and consumers when they are no longer needed to prevent memory leaks.
Example:
producer, err := kafka.NewProducer(&kafka.ConfigMap{"bootstrap.servers": "localhost:9092"})
defer producer.Close() // Ensure cleanup on exit
2. Error Handling
Kafka operations can fail due to transient network issues, authentication errors, or schema mismatches. Implementing a robust error-handling strategy ensures reliability:
- Monitor
Events()
Channel: Continuously check for delivery reports and errors. Unhandled errors can lead to silent failures. - Retry Mechanism: Implement exponential backoff for failed message deliveries to avoid overwhelming the Kafka cluster.
- Dead-Letter Queues (DLQ): Route problematic messages to a DLQ for later analysis rather than discarding them.
- Log Errors with Context: Use structured logging to capture detailed error messages, including timestamps, message keys, and broker responses.
Example:
go func() {
for e := range producer.Events() {
switch ev := e.(type) {
case *kafka.Message:
if ev.TopicPartition.Error != nil {
log.Printf("Delivery failed: %v", ev.TopicPartition.Error)
} else {
log.Printf("Message delivered to %v", ev.TopicPartition)
}
}
}
}()
3. Batch Processing
Batch processing improves Kafka performance by reducing the number of network requests and increasing message throughput. To optimize it:
- Tune
linger.ms
: Settinglinger.ms
to a non-zero value allows Kafka producers to collect multiple messages before sending them in a batch. - Adjust
batch.size
: Increasingbatch.size
optimizes network usage by sending more data per request. - Use Asynchronous Processing: Instead of waiting for each message to be acknowledged before sending the next, leverage Go’s goroutines to process batches concurrently.
- Monitor Batch Latency: Ensure batch sizes do not introduce excessive latency, especially for time-sensitive applications.
Example:
producer, _ := kafka.NewProducer(&kafka.ConfigMap{
"bootstrap.servers": "localhost:9092",
"linger.ms": 10,
"batch.size": 16384,
})
4. Schema Validation
Schemas ensure data consistency across Kafka topics, preventing data corruption and compatibility issues. Follow these best practices:
- Use Confluent Schema Registry: Register and enforce Avro, JSON, or Protobuf schemas to maintain structured data.
- Enable Compatibility Modes: Set compatibility levels (
BACKWARD
,FORWARD
,FULL
) to control schema evolution without breaking existing consumers. - Validate Schema Before Deployment: Before pushing changes, test schema compatibility with existing data pipelines.
- Handle Missing or Incorrect Schemas: Implement proper error handling when consumers receive messages with unexpected schema versions.
Example:
srClient := schemaregistry.NewClient(schemaregistry.NewConfig("https://sr-endpoint.confluent.cloud"))
serializer := NewAvroSerializer(srClient, 5) // Schema ID 5
5. Logging & Metrics
Comprehensive logging and monitoring are crucial for diagnosing Kafka performance issues and debugging errors efficiently. Best practices include:
- Structured Logging: Use a structured logging framework (e.g., Logrus, Zap) to log producer/consumer activity in a readable format.
- Monitor Message Throughput: Track producer and consumer metrics such as messages per second, lag, and consumer group rebalancing.
- Use Prometheus and Grafana: Integrate these tools to visualize Kafka health, lag trends, and broker load.
- Alerting and Monitoring: Set up alerts for critical issues such as high consumer lag, broker downtime, or frequent rebalances.
Example:
log := logrus.New()
log.WithFields(logrus.Fields{
"topic": "my-topic",
"status": "delivered",
}).Info("Message successfully sent")
Following these best practices ensures a scalable, reliable, and high-performance Kafka integration in your Go applications.
Real-World Use Cases
- IoT Data Pipelines: Stream sensor data efficiently using Go's goroutines.
- Fraud Detection Systems: Analyze transaction data in real-time with Kafka Streams.
- Microservices Communication: Decouple services using an event-driven architecture.
- Log Aggregation: Collect and process logs from distributed applications.
Confluent Kafka Go vs. Other Kafka Clients
| Feature | Confluent Kafka Go | Sarama (Community) |
|-----------------|--------------------|---------------------|
| Schema Registry Support | ✅ Native | ❌ Requires Plugins |
| Exactly-Once Semantics | ✅ Fully Supported | ✅ Partial Support |
| Cloud Integration | ✅ Confluent Cloud Ready | ❌ Self-Managed Only |
| Security Features | ✅ Advanced (SASL, SSL) | ✅ Basic |
Troubleshooting Tips
Message Delivery Failures
If messages are not being delivered to Kafka, check the deliveryChan
for error messages such as _MSG_TIMED_OUT
. This typically happens due to network issues, broker unavailability, or incorrect producer configurations. To troubleshoot:
- Verify that the Kafka brokers are reachable and running.
- Ensure that the producer is correctly configured with the appropriate
bootstrap.servers
. - Increase
request.timeout.ms
if needed to handle transient network latencies. - Enable logging to capture detailed error messages and debug the issue.
Schema Registry Errors
If you encounter issues with Schema Registry, such as deserialization errors or schema mismatches, verify that the compatibility modes (BACKWARD, FORWARD, FULL) are correctly set. Common issues and fixes include:
- Incompatible Schema Changes: Ensure that new schema versions are compatible with existing data. Use Schema Registry's
compatibility
mode to enforce validation. - Invalid Schema IDs: Check if the producer/consumer is using the correct schema ID when serializing/deserializing messages.
- Authentication Issues: If using Confluent Cloud, confirm that API keys and authentication credentials are correctly configured.
- Schema Not Found: Ensure that the Schema Registry endpoint is reachable and the required schema is registered before producing messages.
Consumer Lag Issues
Consumer lag occurs when messages accumulate in Kafka topics because consumers are not processing them fast enough. This can lead to stale data processing and potential system overload. To mitigate consumer lag:
- Tune
max.poll.interval.ms
: Increase this value to allow consumers more time to process messages before they are removed from the group. - Optimize Parallel Processing: Utilize Go’s concurrency model to handle messages efficiently in multiple goroutines.
- Adjust Partition Assignments: Ensure an even distribution of partitions across consumers in a consumer group to balance the load.
- Monitor Consumer Group Lag: Use tools like Kafka’s
kafka-consumer-groups.sh
or Confluent Control Center to track lag and take corrective actions.
Performance Tuning
Fine-tuning producer and consumer configurations can optimize Kafka performance. Consider the following adjustments:
- Optimize
linger.ms
andbatch.size
: Increaselinger.ms
to allow batching of messages, reducing the number of requests and improving throughput. Similarly, adjustbatch.size
to fine-tune the number of messages sent per batch. - Use Compression: Enable message compression (
compression.type
set togzip
orsnappy
) to reduce network overhead. - Adjust Fetch Sizes: Modify
fetch.min.bytes
andfetch.max.wait.ms
for consumers to optimize data retrieval rates. - Scale Producers and Consumers: If throughput is a concern, consider scaling the number of producers and consumers to handle larger workloads efficiently.
- Monitor Resource Utilization: Regularly check CPU, memory, and disk usage on Kafka brokers to ensure optimal performance.
Resources to Master Confluent Kafka Go
- Official Documentation: Confluent Kafka Go GitHub
- Tutorials & Guides: Confluent Developer Portal’s Go-focused Kafka tutorials.
- Community Support: Join Confluent Community Slack (#go-client channel) for discussions and help.
Conclusion
Confluent Kafka Go empowers developers to build highly efficient, real-time data pipelines using Golang. Whether you’re handling large-scale event streaming, microservices communication, or real-time analytics, this library provides the essential tools and integrations required for success.
To get started, set up a test environment, explore key features, and optimize your implementation by leveraging advanced Kafka capabilities like Exactly-Once Semantics and Schema Registry.