Gin, Echo, and Fiber Compared: Which One Should You Choose?
Real-World Performance Comparison of Golang Gin, Echo, and Fiber Frameworks with Response Time Benchmarks
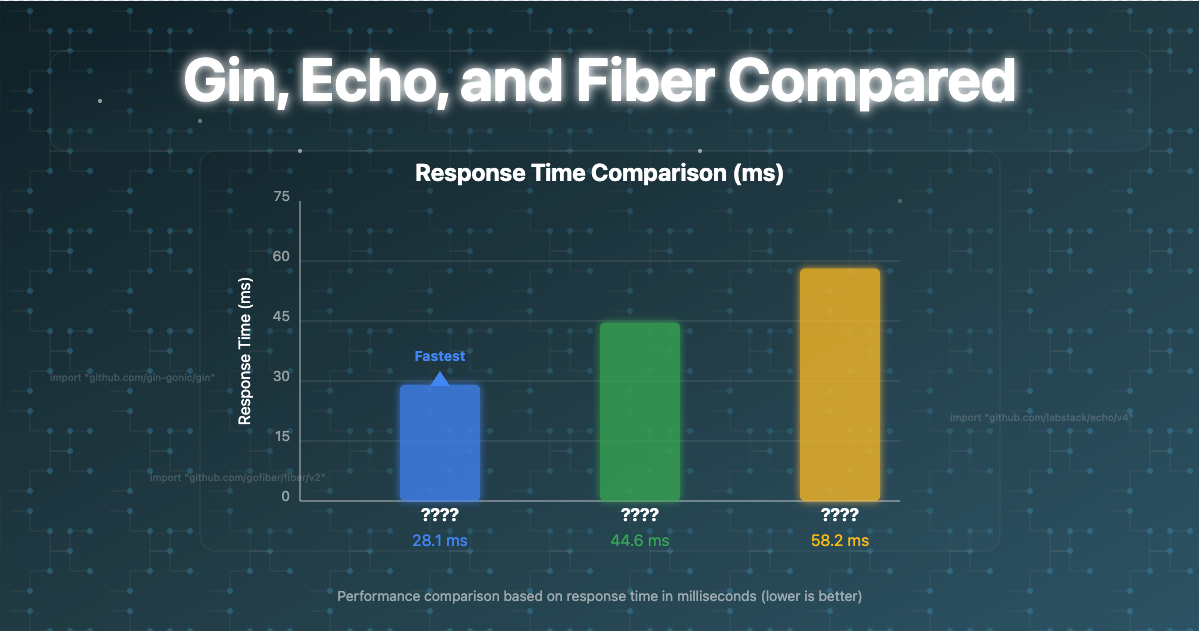
I was facing a problem while choosing between the Golang frameworks for my next project. So I decided to test each of them to find the best one to process my request faster. I have created the same program in 3 different golang web frameworks to test and provide a comparison between them. I have chosen the Gin, Echo, and Fiber golang web frameworks.
Test Setup
To test the application, we need to create three applications with a simple HTTP GET request listening on port 8080 for GIN, Echo. and Fiber. I have used the following command to create the projects
mkdir golang-test
cd golang-test
mkdir gin
cd gin
go mod init gin-test
cd ../
mkdir echo
cd echo
go mod init echo-test
cd ../
mkdir fiber
cd fiber
go mod init fibcer-test
create directories for golang web framework test
After successfully creating and implementing the project, we have to create the main.Go to the file in each folder to create a simple HTTP GET server. Here is the sample code for each of them -
gin.main.go
package main
import (
"log"
"time"
"github.com/gin-gonic/gin"
)
func main() {
r := gin.Default()
// Middleware to measure time
r.Use(func(c *gin.Context) {
start := time.Now()
c.Next()
elapsed := time.Since(start)
log.Printf("Gin: %s %s took %s\n", c.Request.Method, c.Request.URL.Path, elapsed)
})
r.GET("/hello", func(c *gin.Context) {
c.JSON(200, gin.H{"message": "Hello, World!"})
})
r.Run(":8080")
}
Golang gin http with took time middleware
echo/main.go
package main
import (
"log"
"time"
"github.com/labstack/echo/v4"
)
func main() {
e := echo.New()
// Custom timing middleware
e.Use(func(next echo.HandlerFunc) echo.HandlerFunc {
return func(c echo.Context) error {
start := time.Now()
err := next(c)
elapsed := time.Since(start)
log.Printf("Echo: %s %s took %s\n", c.Request().Method, c.Request().URL.Path, elapsed)
return err
}
})
e.GET("/hello", func(c echo.Context) error {
return c.JSON(200, map[string]string{"message": "Hello, World!"})
})
e.Logger.Fatal(e.Start(":8080"))
}
Golang echo http with took time middleware
fiber/main.go
// main.go (Fiber)
package main
import (
"log"
"time"
"github.com/gofiber/fiber/v2"
)
func main() {
app := fiber.New()
// Middleware to measure time
app.Use(func(c *fiber.Ctx) error {
start := time.Now()
err := c.Next()
elapsed := time.Since(start)
log.Printf("Fiber: %s %s took %s\n", c.Method(), c.Path(), elapsed)
return err
})
app.Get("/hello", func(c *fiber.Ctx) error {
return c.JSON(fiber.Map{"message": "Hello, World!"})
})
log.Fatal(app.Listen(":8080"))
}
Golang fiber http with took time middleware
Now we have all http servers ready to serve the http get request at http://localhost:8080/hello
Response
{
"message": "Hello, World!"
}
Start testing
To test by simulating 1000 requests, I used a bash script to send the requests. You can create this file by pasting the following content -
run.sh
#!/bin/bash
URL="http://localhost:8080/hello"
COUNT=1000
echo "Sending $COUNT requests to $URL..."
for ((i = 1; i <= COUNT; i++)); do
curl -s -o /dev/null "$URL"
done
After that, we have to change the permission of this run.sh
file to execute it.
chmod +x ./run.sh
Now, we are ready to test. Use the following command to run each HTTP server one by one and execute the run.sh file to send 1000 requests to each server
# run gin server
cd gin && go run main.go
# run echo server
cd echo && go run main.go
# run fiber server
cd fiber && go run main.go
# run bash file
./run.sh
Results
I am attaching the screenshots for each of the http server response below -
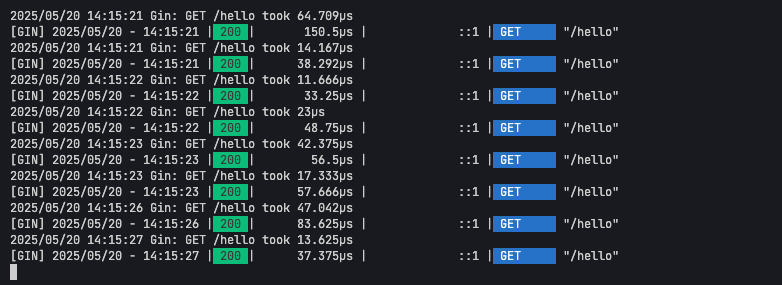
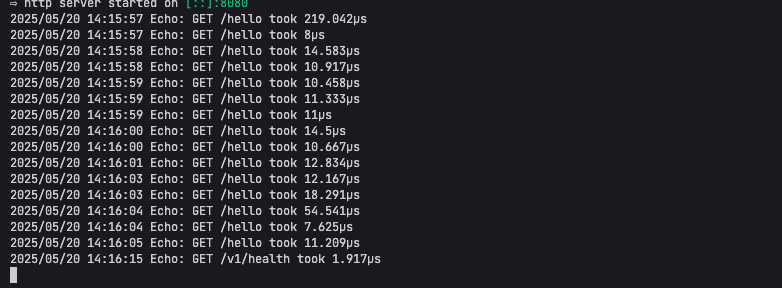
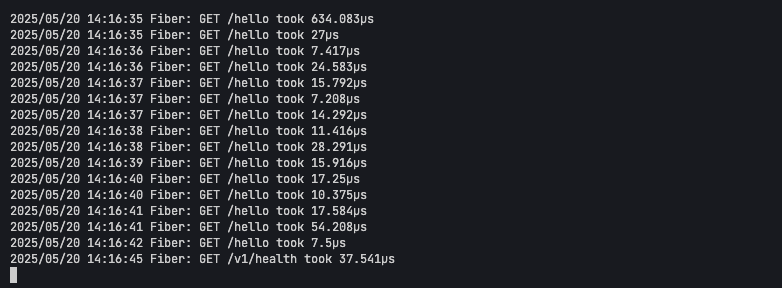
Average execution time
After simulating the test, I have the average execution time for each framework, which is -
GIN - 44.6 ms
Echo - 28.1 ms
Fiber - 58.2 ms

So here we have the results of getting started with your next golang web framework.
Conclusion
I have shown the results for each of them, and here are my final thoughts for choosing the Golang framework for your next web project -
If you want the easiest, then use - Gin
If you are from an express.js background, then use - Fiber
Thank you for reading, feel free to provide your thoughts