Golang and AI
People don’t usually pick Go first for AI, but it can still be useful.
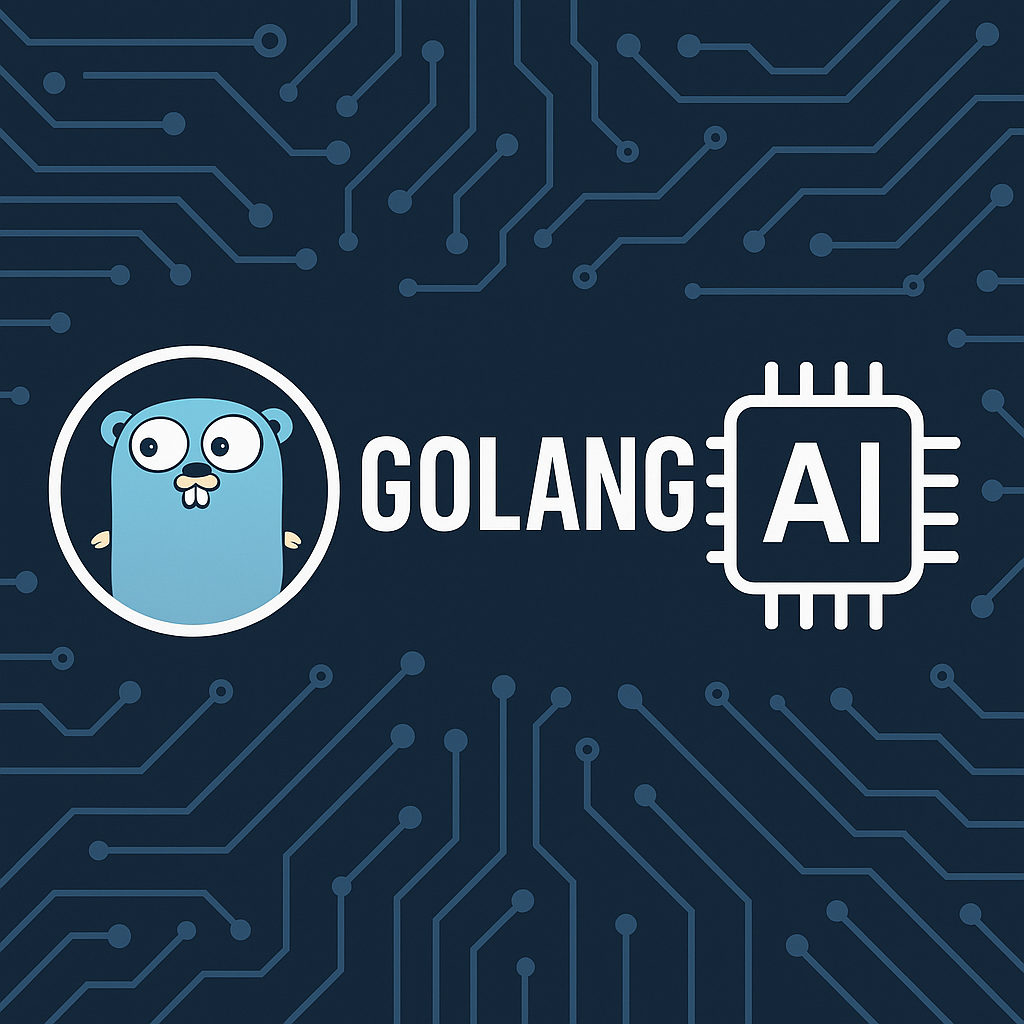
Golang (also called Go) is a compiled language made by Google. It’s known for being simple, fast, and good at handling many tasks at once. People often use Go to build backend services, APIs, and tools that need high performance.
Go is not a top choice for artificial intelligence (AI) or machine learning (ML). Most AI work is done in Python. But Go still has a place. It can help run AI models in production, handling data, or connecting systems.
Is Go Good for AI?
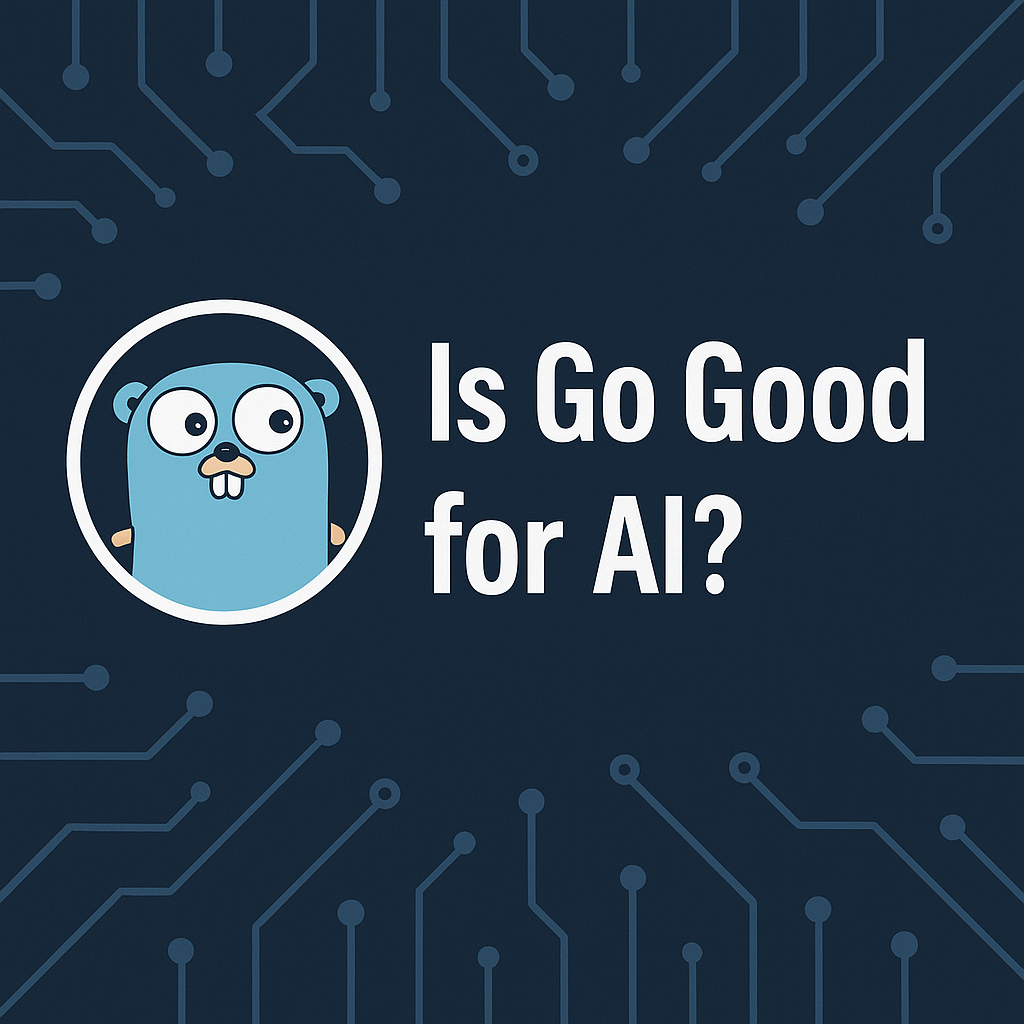
Go is not made for AI the way Python is. Python has thousands of libraries, tutorials, and ready-made models. It’s easy to experiment and train models with Python. Most ML engineers and data scientists use Python every day.
Go doesn’t offer that level of support, but it has other strengths:
- Fast Execution: Go is compiled. So code runs faster than Python in many cases. This helps when building APIs or services that need low response times.
- Concurrency: Go has goroutines. They make it simple to run many tasks at the same time. This is useful for processing lots of data or handling requests in parallel.
- Easy Deployment: Go builds a single binary. That means you don’t need virtual environments or extra setup. This makes it easier to deploy services that use AI models.
- Stability: Go has strict typing and fewer moving parts. Your services tend to be more stable and easier to debug.
Common Use Cases
1. Serving Models
This is the most common way Go is used with AI. You train your model in Python (using TensorFlow, PyTorch, etc.), export it (like a .pb, .onnx, or .pt file), and then use Go to serve it in a web API or microservice.
You don’t run the training in Go. You just use Go to load the saved model and run predictions.
Example:
- A Python model is trained to detect spam emails.
- It is saved as an ONNX file.
- A Go service loads that ONNX file and serves a /predict API.
2. Pre- and Post-processing
AI systems often need to clean input data, convert formats, or reshape results. Go is good at handling text, JSON, images, or other formats. You can do this work in Go before and after model calls.
Example:
- A user uploads an image.
- Go resizes and normalizes the image.
- The image is sent to a Python model.
- The result is sent back to Go, and formatted as a user-readable message.
3. Integration with Systems
In real-world apps, AI is just one part. You also need to store data, send notifications, run batch jobs, or fetch results. Go connects well with databases, queues (like Kafka or Pub/Sub), and APIs.
You can build the whole surrounding system in Go, and just plug in AI when needed.
Libraries in Go for AI
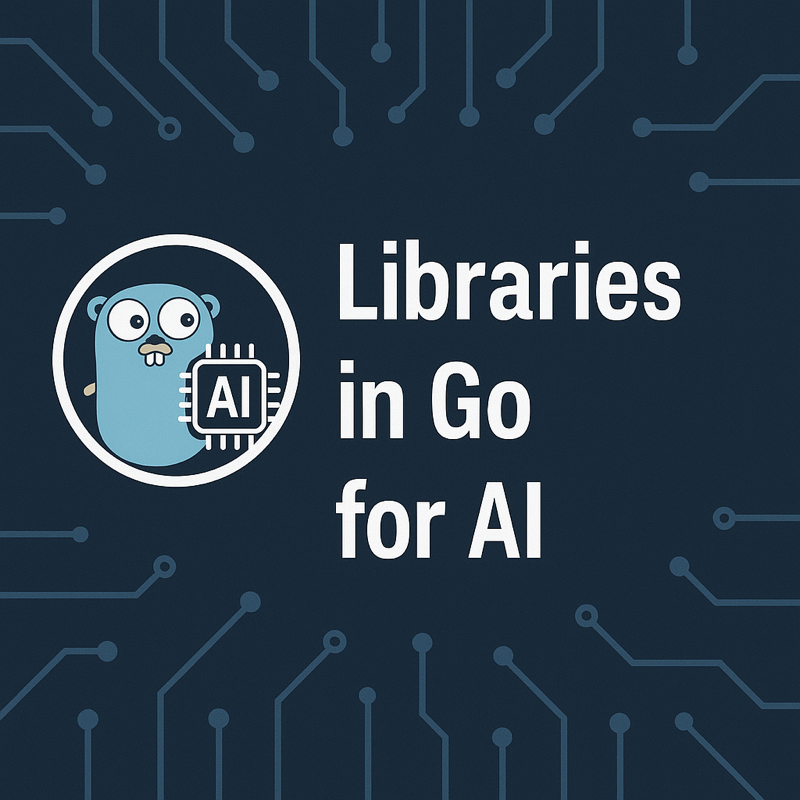
Here are some Go libraries that try to support AI or ML:
1. Gorgonia
Gorgonia is a library for building and running neural networks in Go. It looks like TensorFlow but with Go syntax. You define computation graphs and can build simple deep learning models. It works, but the community is small.
2. Gotch
Gotch is a wrapper around LibTorch, which is the C++ backend of PyTorch. This means you can run PyTorch models using Go. You still need to write some low-level code, but it’s more powerful than Gorgonia.
3. GoLearn
GoLearn is for traditional ML. It supports decision trees, k-nearest neighbors, Naive Bayes, and other basic algorithms. It’s okay for small projects or learning purposes, but not used much in production.
4. onnx-go
This library lets you load ONNX models in Go. ONNX is a common format to save models from different frameworks (PyTorch, TensorFlow, etc.). You can train a model in Python and run it in Go.
When to Use Go in AI
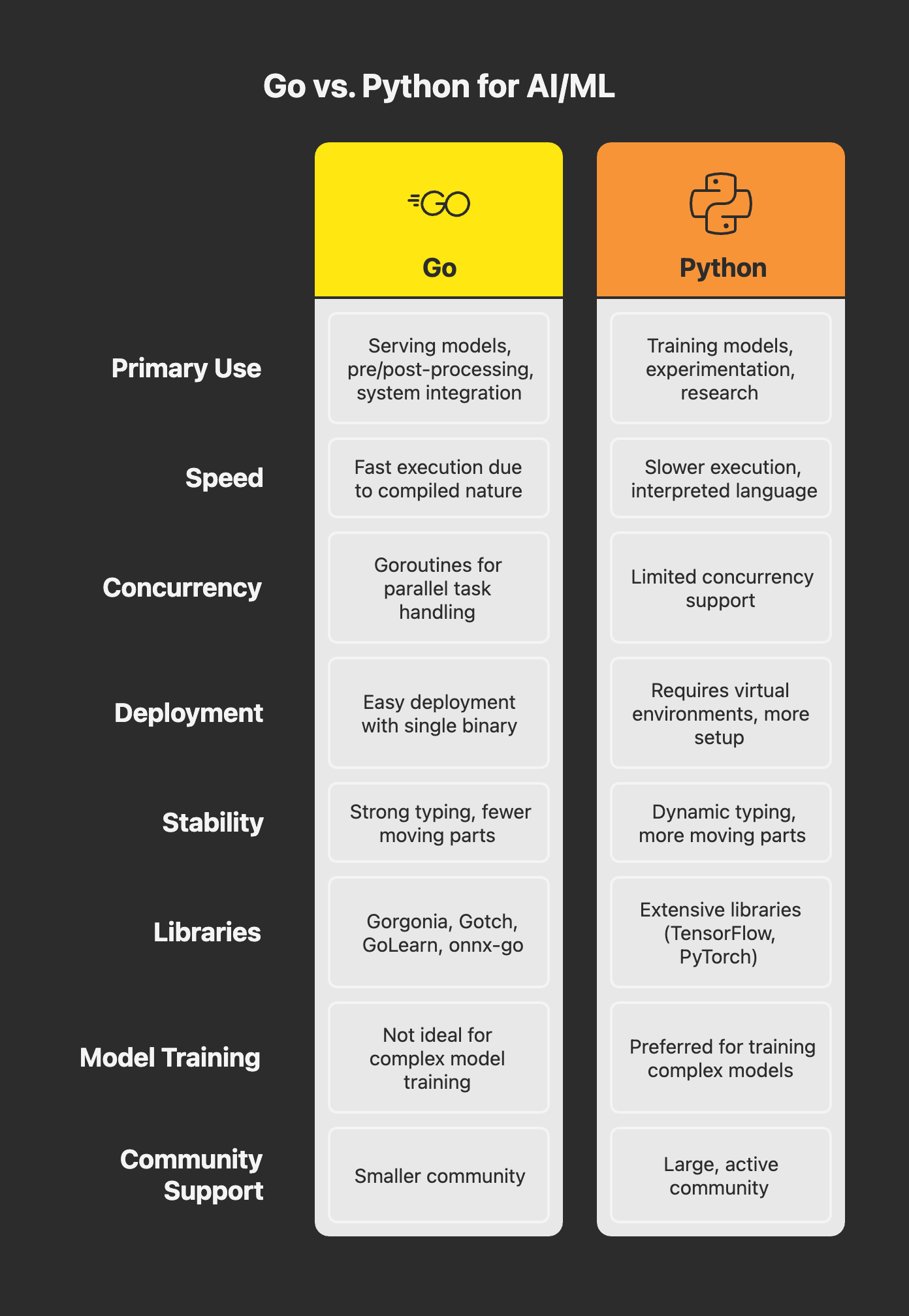
Use Go when:
- Your backend or infrastructure is already written in Go.
- You only need to serve predictions, not train models.
- You care about speed and want a small, self-contained service.
- You want strong typing and fast error checking at compile time.
Don’t use Go when:
- You want to train complex models like deep neural networks.
- You need access to popular ML tools, libraries, or notebooks.
- You’re doing research or rapid experimentation.
- You want community support or many learning resources.
Final Thoughts
Go is not the main language for AI work. But it’s still useful—especially when you want to run trained models fast and reliably. If your app or service is built in Go, it makes sense to keep the AI parts in the same language as much as possible.
But if you’re training models or doing anything experimental, it’s better to stick with Python.