Golang Gin Authentication Middleware Example
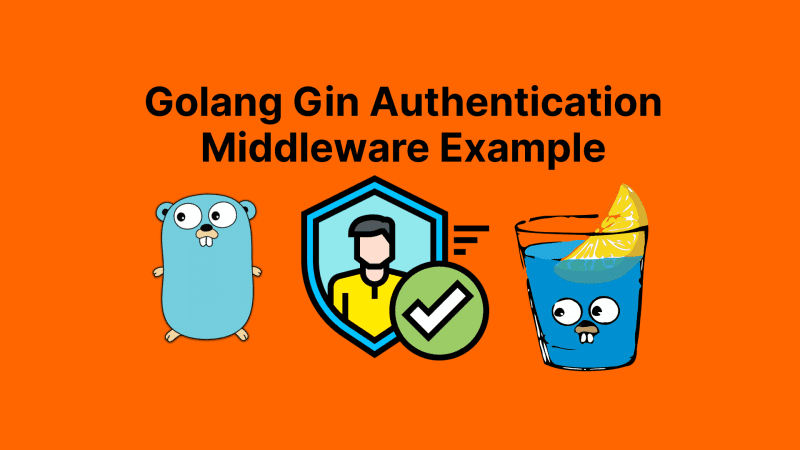
In this article, we will explore how to implement authentication middleware in Golang using the Gin framework. Middleware is a powerful feature that allows you to intercept requests and perform actions before they reach your handlers. We will use JSON Web Tokens (JWT) to secure our APIs.
Setting Up Gin
First, let’s set up a basic Gin application. If you haven’t already, install Gin using the following command:
go get -u github.com/gin-gonic/gin
Create a new file main.go and initialize your Gin application:
package main
import (
"github.com/gin-gonic/gin"
)
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
Implementing JWT Authentication Middleware
To implement JWT authentication, we need to create a middleware function that will validate the token. Let’s start by installing the JWT package:
go get -u github.com/dgrijalva/jwt-go
Now, create a new file middleware.go and add the following code:
package main
import (
"github.com/gin-gonic/gin"
"github.com/dgrijalva/jwt-go"
"net/http"
"strings"
)
func AuthMiddleware() gin.HandlerFunc {
return func(c *gin.Context) {
authHeader := c.GetHeader("Authorization")
if authHeader == "" {
c.JSON(http.StatusUnauthorized, gin.H{"error": "Authorization header required"})
c.Abort()
return
}
tokenString := strings.TrimPrefix(authHeader, "Bearer ")
token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) {
if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok {
return nil, jwt.ErrSignatureInvalid
}
return []byte("your-secret-key"), nil
})
if err != nil || !token.Valid {
c.JSON(http.StatusUnauthorized, gin.H{"error": "Invalid token"})
c.Abort()
return
}
c.Next()
}
}
Securing Routes with Middleware
Now that we have our middleware, let’s secure our routes. Update your main.go file to include the middleware:
package main
import (
"github.com/gin-gonic/gin"
)
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
authorized := r.Group("/")
authorized.Use(AuthMiddleware())
{
authorized.GET("/secure", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "You have access to this secure endpoint!",
})
})
}
r.Run() // listen and serve on 0.0.0.0:8080
}
Testing the Middleware
To test the middleware, you can use a tool like Postman or curl to send requests to your server. Make sure to include the Authorization header with a valid JWT token.
curl -H "Authorization: Bearer your-valid-jwt-token" http://localhost:8080/secure
Conclusion
In this article, we have implemented a simple authentication middleware using Gin and JWT in Golang. This setup allows you to secure your APIs and ensure that only authenticated users can access certain endpoints. Feel free to extend this example by adding more features such as role-based access control or token expiration handling.
Note: Always keep your secret keys secure and never expose them in your code. Consider using environment variables or a secure vault to manage sensitive information.