GraphQL and Golang Integration Tutorial for Beginners
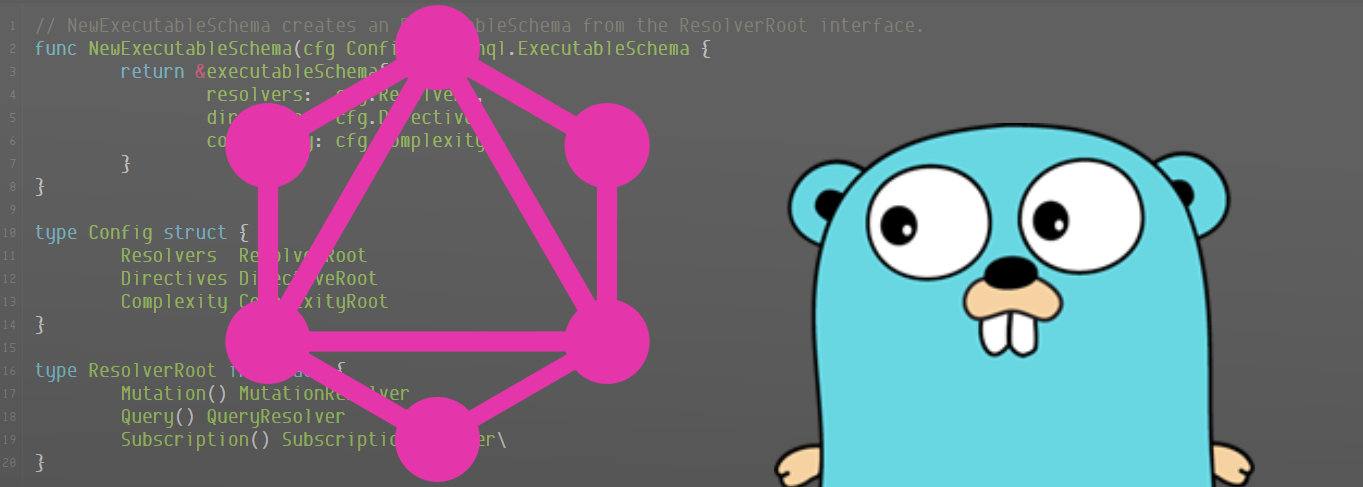
GraphQL is a query language for APIs that allows for more flexible and efficient data retrieval, while Golang is a modern programming language developed by Google. Integrating GraphQL with Golang can help developers build scalable and high-performance APIs. In this tutorial, we will explore the basics of GraphQL and Golang integration, and provide a step-by-step guide on how to get started.
What is GraphQL
GraphQL is a query language for APIs that allows clients to specify exactly what data they need, and receive only that data in response. This approach reduces the amount of data transferred over the network, making it more efficient and scalable. GraphQL also provides a strong typing system, which helps catch errors at compile-time rather than runtime.
Key Features of GraphQL
Some of the key features of GraphQL include:
- Schema-driven development: GraphQL APIs are defined using a schema, which provides a clear and concise definition of the available types and fields.
- Query language: GraphQL provides a query language that allows clients to specify what data they need, and receive only that data in response.
- Strong typing: GraphQL has a strong typing system, which helps catch errors at compile-time rather than runtime.
- Real-time updates: GraphQL provides support for real-time updates, allowing clients to receive updates as soon as new data is available.
What is Golang
Golang, also known as Go, is a modern programming language developed by Google. It is designed to be simple, efficient, and easy to use, making it a popular choice for building scalable and high-performance systems. Golang has a number of features that make it well-suited for building APIs, including:
- Concurrency support: Golang has built-in support for concurrency, making it easy to write programs that can handle multiple tasks simultaneously.
- Goroutines: Golang's goroutine scheduling algorithm allows for efficient and lightweight concurrency.
- Channels: Golang's channel system provides a safe and efficient way to communicate between goroutines.
Key Features of Golang
Some of the key features of Golang include:
- Simple syntax: Golang has a simple and concise syntax, making it easy to learn and use.
- Fast execution: Golang programs are compiled to machine code, making them fast and efficient.
- Reliable: Golang has a strong focus on reliability, with features such as memory safety and data race detection.
Integrating GraphQL with Golang
Setting up the Project
To integrate GraphQL with Golang, we will need to set up a new Golang project and install the necessary dependencies. We will use the github.com/graphql-go/graphql
package to provide GraphQL support, and the github.com/gorilla/mux
package to provide HTTP routing support.
Defining the GraphQL Schema
The first step in integrating GraphQL with Golang is to define the GraphQL schema. The schema defines the available types and fields, and provides a clear and concise definition of the API. We will define a simple schema that includes a User
type with id
, name
, and email
fields.
Implementing Resolvers
Once the schema is defined, we need to implement resolvers for each field. Resolvers are functions that return the data for a given field, and are used to fetch data from the underlying data storage system. We will implement resolvers for the User
type, using a simple in-memory data storage system.
Creating the GraphQL Server
With the schema and resolvers defined, we can create the GraphQL server. We will use the github.com/graphql-go/graphql
package to create a new GraphQL server, and the github.com/gorilla/mux
package to provide HTTP routing support.
Example Code
main.go
package main
import (
"encoding/json"
"fmt"
"log"
"net/http"
"github.com/graphql-go/graphql"
"github.com/gorilla/mux"
)
// Define the User type
var userType = graphql.NewObject(graphql.ObjectConfig{
Name: "User",
Fields: graphql.Fields{
"id": &graphql.Field{
Type: graphql.Int,
},
"name": &graphql.Field{
Type: graphql.String,
},
"email": &graphql.Field{
Type: graphql.String,
},
},
})
// Define the root query
var rootQuery = graphql.NewObject(graphql.ObjectConfig{
Name: "Query",
Fields: graphql.Fields{
"user": &graphql.Field{
Type: userType,
Args: graphql.FieldConfigArgument{
"id": &graphql.ArgumentConfig{
Type: graphql.Int,
},
},
Resolve: func(p graphql.ResolveParams) (interface{}, error) {
// Implement the resolver for the user field
id, ok := p.Args["id"].(int)
if !ok {
return nil, fmt.Errorf("invalid id")
}
// Fetch the user data from the underlying data storage system
user := &User{
ID: id,
Name: "John Doe",
Email: "john@example.com",
}
return user, nil
},
},
},
})
// Define the GraphQL schema
var schema, _ = graphql.NewSchema(graphql.SchemaConfig{
Query: rootQuery,
})
func main() {
// Create a new GraphQL server
srv := &http.Server{
Addr: ":8080",
Handler: mux.NewRouter().PathPrefix("/graphql").HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
// Handle GraphQL requests
result := graphql.Do(graphql.Params{
Schema: schema,
RequestString: r.URL.Query().Get("query"),
})
json.NewEncoder(w).Encode(result)
}),
}
log.Fatal(srv.ListenAndServe())
}
type User struct {
ID int
Name string
Email string
}
Conclusion
In this tutorial, we have explored the basics of GraphQL and Golang integration, and provided a step-by-step guide on how to get started. We have defined a simple GraphQL schema, implemented resolvers, and created a GraphQL server using the github.com/graphql-go/graphql
package. With this knowledge, you can start building your own GraphQL APIs using Golang, and take advantage of the flexibility and efficiency that GraphQL provides.