Idiomatic Go Writing Mistakes Beginners Should Avoid
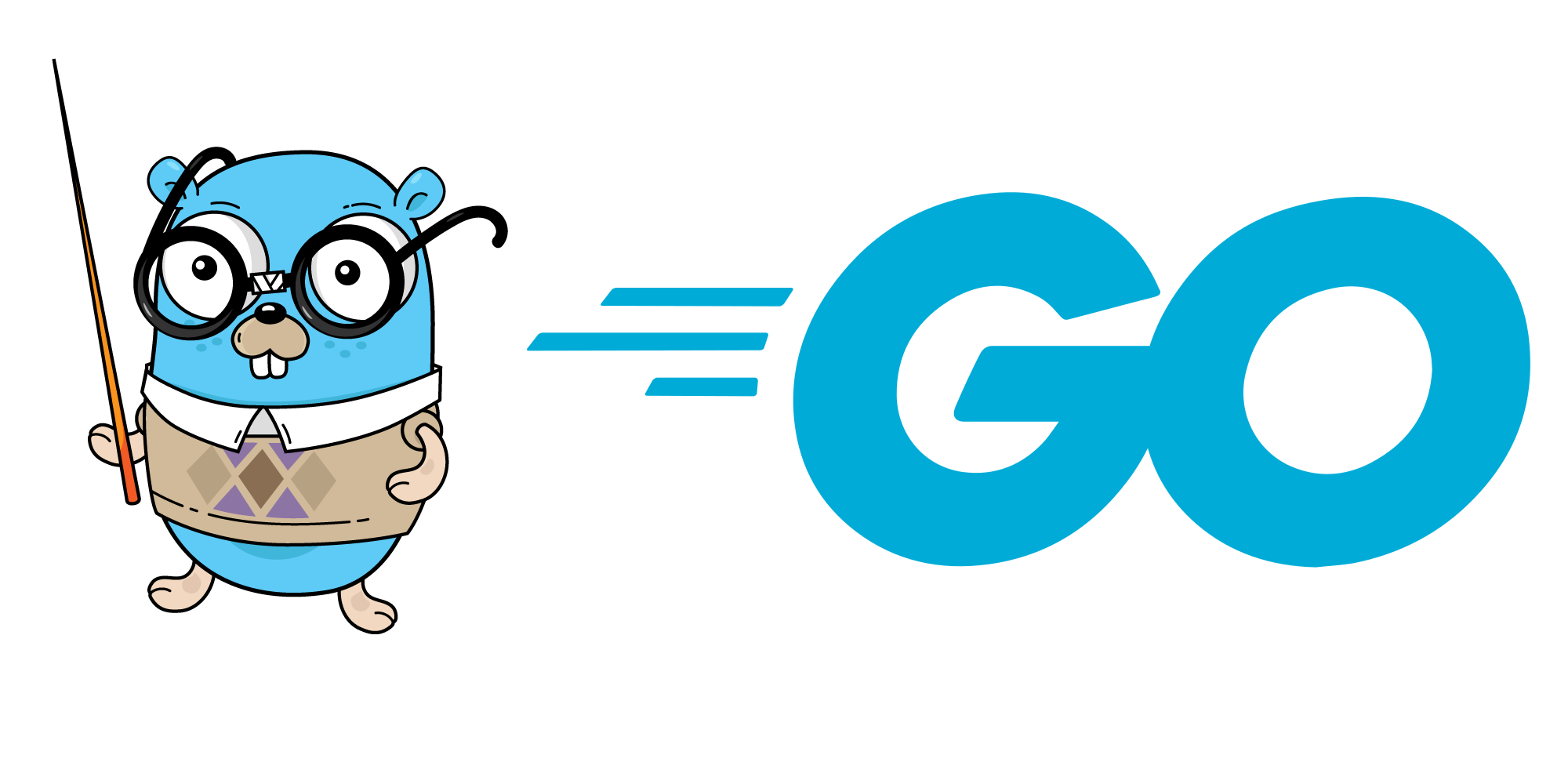
Go, also known as Golang, is a statically typed, compiled, designed to be concurrent and garbage-collected programming language developed by Google. As a beginner in Go, it is essential to write idiomatic code to ensure readability, maintainability, and performance. Idiomatic code is code that follows the common practices and conventions of the language. In this post, we will discuss some common mistakes beginners make when writing Go code and how to avoid them.
Error Handling
Error handling is a crucial aspect of writing robust and reliable code. In Go, errors are handled using the err
type, which is a built-in interface. A common mistake beginners make is ignoring errors or not handling them properly.
func main() {
file, _ := os.Open("example.txt")
// ...
}
In the above example, the error returned by os.Open
is ignored. This can lead to unexpected behavior and make it difficult to debug issues. Instead, errors should be handled properly using an if
statement or a switch statement.
func main() {
file, err := os.Open("example.txt")
if err != nil {
log.Fatal(err)
}
// ...
}
Goroutine Management
Goroutines are lightweight threads in Go that can run concurrently with the main program flow. A common mistake beginners make is not managing goroutines properly.
func main() {
go func() {
// ...
}()
// ...
}
In the above example, a goroutine is started, but it is not managed properly. This can lead to resource leaks and unexpected behavior. Instead, goroutines should be managed using channels or wait groups.
func main() {
var wg sync.WaitGroup
wg.Add(1)
go func() {
// ...
wg.Done()
}()
wg.Wait()
// ...
}
Channel Usage
Channels are a fundamental concept in Go that allow goroutines to communicate with each other. A common mistake beginners make is using channels incorrectly.
func main() {
ch := make(chan int)
ch <- 1
// ...
}
In the above example, a channel is created, but it is not buffered. This can lead to deadlocks and unexpected behavior. Instead, channels should be buffered or used with a select statement.
func main() {
ch := make(chan int, 1)
ch <- 1
// ...
}
Slice and Array Usage
Slices and arrays are used to store collections of data in Go. A common mistake beginners make is using slices and arrays incorrectly.
func main() {
arr := [5]int{1, 2, 3, 4, 5}
slice := arr[1:3]
// ...
}
In the above example, a slice is created from an array, but it is not used correctly. This can lead to unexpected behavior and make it difficult to debug issues. Instead, slices and arrays should be used with the len
and cap
functions.
func main() {
arr := [5]int{1, 2, 3, 4, 5}
slice := arr[1:3]
fmt.Println(len(slice)) // prints 2
fmt.Println(cap(slice)) // prints 4
// ...
}
Map Usage
Maps are used to store key-value pairs in Go. A common mistake beginners make is using maps incorrectly.
func main() {
m := make(map[string]int)
m["one"] = 1
// ...
}
In the above example, a map is created, but it is not used correctly. This can lead to unexpected behavior and make it difficult to debug issues. Instead, maps should be used with the range
keyword.
func main() {
m := make(map[string]int)
m["one"] = 1
for k, v := range m {
fmt.Println(k, v)
}
// ...
}
Struct and Interface Usage
Structs and interfaces are used to define custom data types in Go. A common mistake beginners make is using structs and interfaces incorrectly.
func main() {
type Person struct {
name string
age int
}
p := Person{name: "John", age: 30}
// ...
}
In the above example, a struct is created, but it is not used correctly. This can lead to unexpected behavior and make it difficult to debug issues. Instead, structs and interfaces should be used with the .
notation.
func main() {
type Person struct {
name string
age int
}
p := Person{name: "John", age: 30}
fmt.Println(p.name) // prints John
fmt.Println(p.age) // prints 30
// ...
}
Best Practices
In addition to avoiding common mistakes, there are several best practices that beginners should follow when writing Go code. These include:
- Using meaningful variable names
- Using comments to explain complex code
- Using functions to organize code
- Using testing to ensure code correctness
- Using linters to ensure code style consistency
By following these best practices and avoiding common mistakes, beginners can write idiomatic Go code that is readable, maintainable, and performant.
Conclusion of Best Practices
In conclusion, writing idiomatic Go code requires a combination of knowledge of the language, best practices, and common mistakes to avoid. By following the guidelines outlined in this post, beginners can write high-quality Go code that is efficient, readable, and easy to maintain.
Common Mistakes Recap
To recap, some common mistakes beginners make when writing Go code include:
- Ignoring errors or not handling them properly
- Not managing goroutines properly
- Using channels incorrectly
- Using slices and arrays incorrectly
- Using maps incorrectly
- Using structs and interfaces incorrectly
By avoiding these common mistakes and following best practices, beginners can write idiomatic Go code that is efficient, readable, and easy to maintain.
Additional Resources
For more information on writing idiomatic Go code, beginners can refer to the official Go documentation, as well as various online resources and tutorials. Some recommended resources include:
These resources provide a comprehensive overview of the Go language, including its syntax, semantics, and best practices. By studying these resources and practicing writing idiomatic Go code, beginners can become proficient in the language and write high-quality code.