JavaScript List (JS List) - Everything You Need to Know About Arrays in JS
JavaScript doesn’t have a dedicated “list” type; instead, it uses arrays to store ordered collections of data.
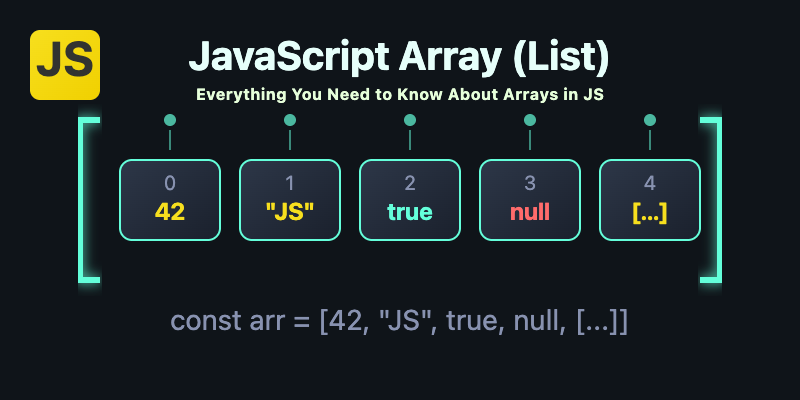
When programmers say “JavaScript list” or “JS list”, they are most often referring to the JavaScript array. Unlike in other programming languages, such as Python, where the term list refers to a specific data structure, JavaScript does not have a separate List type. Instead, all list-like features — storing items sequentially, index-based access, and addition or removal of items — are performed using arrays.
Still, the term list is informally employed within the JavaScript world. One can easily come across JavaScript tutorials, discussions on Stack Overflow, or documentation that refer to “JS list” when they are talking about an array. Such inconsistencies can be problematic to new learners coming from different programming environments, or searching for information online using phrases like “JavaScript list”.
In this article, the term “JavaScript list” or “JS list” refers to an array, which is an object type in JavaScript that manages ordered sets of data. The features of JavaScript, such as lists of user names, product items, or even a set of numbers, can all be stored in arrays. To put it simply, arrays are the core of JavaScript code because they are widely utilized in JavaScript and help improve the efficiency and cleanliness of the code.
Let’s explore what else these collections provide, how you can create and modify them, and what these collections can be tailored to fit.
What is a JavaScript List (JS List)?
In simple terms, a JavaScript list — or more accurately, a JavaScript array — is a way to store multiple values in a single variable. Think of it as a flexible container that holds items in a specific order, and each item is accessible by a number called an index, starting from 0.
let fruits = ["apple", "banana", "mango"];
In the example above, fruits[0] gives you "apple", fruits[1] is "banana", and so on. You can store anything in an array — numbers, strings, objects, even other arrays.
How to Create a JavaScript List
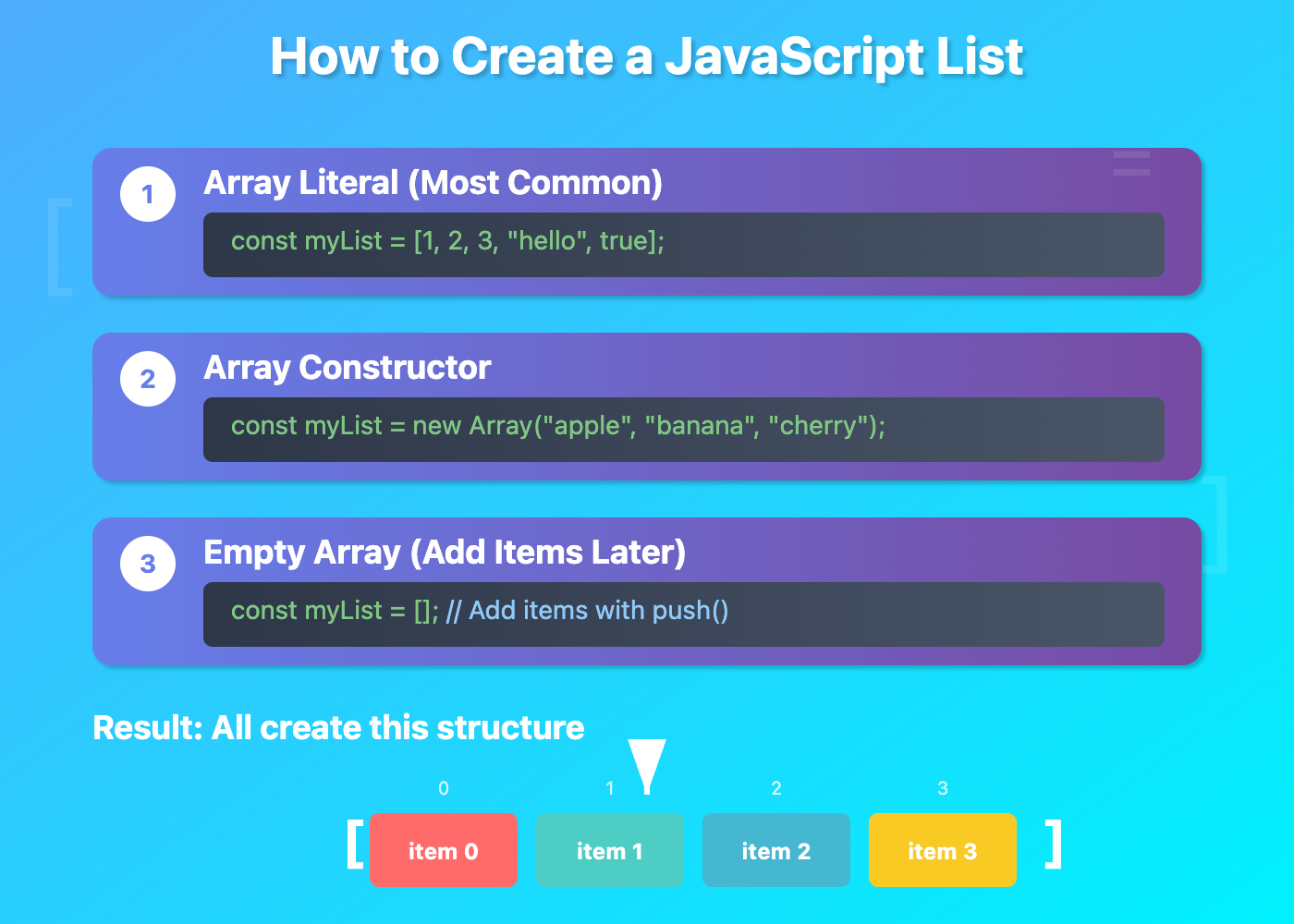
Creating a list in JavaScript (technically, an array) is very straightforward. JavaScript offers a couple of ways to do this, depending on your use case. Let’s look at the most common and practical approaches below.
Using Array Literals (Recommended)
let colors = ["red", "green", "blue"];
In this example, colors is a list containing three string elements. Each item in the list is stored in a specific position, starting from index 0.
console.log(colors[0]); // "red"
console.log(colors[2]); // "blue"
You can also create an empty list and add items to it later:
let tasks = [];
tasks.push("Write blog");
tasks.push("Fix bug");
Using the Array Constructor
Another way to create a list is by using the Array constructor. This method is generally less preferred unless you have a specific reason to use it.
let numbers = new Array(10, 20, 30);
Or to create an empty array of a fixed length:
let emptySlots = new Array(5); // Creates an array with 5 empty items
However, note that if you pass a single number to the constructor, JavaScript treats it as the array’s length — not an element.
let wrongWay = new Array(5);
console.log(wrongWay); // [ <5 empty items> ]
So unless you’re intentionally creating a sparse array, stick to array literals.
Creating Lists from Strings, Sets, or Other Arrays
You can also convert other data types into lists using built-in methods:
let str = "hello";
let chars = Array.from(str); // ['h', 'e', 'l', 'l', 'o']
Or convert a Set to a list:
let uniqueNumbers = new Set([1, 2, 3]);
let numberList = Array.from(uniqueNumbers); // [1, 2, 3]
Accessing and Modifying List Items in JavaScript
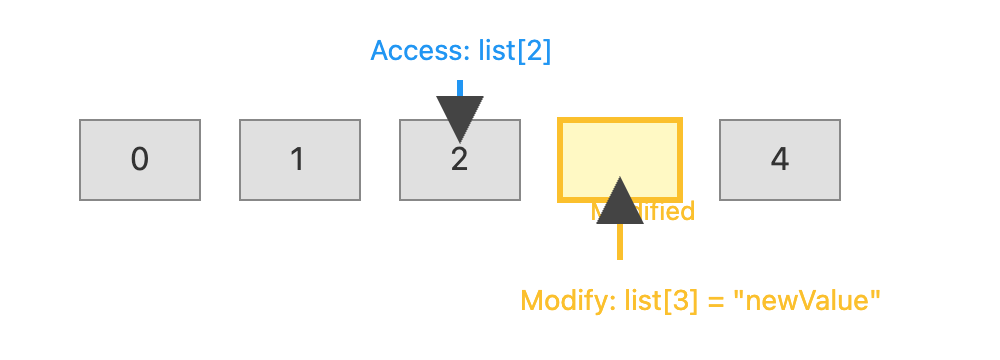
Once you’ve created a list (array) in JavaScript, the next step is learning how to access, change, add, and remove items. These are basic operations that you’ll use almost every time you work with lists.
Accessing Items by Index
Each item in a JavaScript list has a position, or index, starting from 0.
let fruits = ["apple", "banana", "mango"];
console.log(fruits[0]); // "apple"
console.log(fruits[2]); // "mango"
If you try to access an index that doesn’t exist, JavaScript returns undefined:
console.log(fruits[5]); // undefined
Modifying Items
You can change the value of any element by assigning a new value to its index:
fruits[1] = "orange";
console.log(fruits); // ["apple", "orange", "mango"]
Adding Items
There are several ways to add new items to a JavaScript list, depending on where you want to insert the element.
Add to the End:
push()
fruits.push("grape");
console.log(fruits); // ["apple", "orange", "mango", "grape"]
Add to the Beginning:
unshift()
fruits.unshift("kiwi");
console.log(fruits); // ["kiwi", "apple", "orange", "mango", "grape"]
Add at a Specific Position:
splice()
fruits.splice(2, 0, "pineapple");
// Insert at index 2, delete 0 items
console.log(fruits);
Removing Items
You can also remove items in several ways:
Remove from the End:
pop()
fruits.pop(); // removes "grape"
Remove from the Beginning:
shift()
fruits.shift(); // removes "kiwi"
Remove from a Specific Index:
splice()
fruits.splice(1, 2);
// From index 1, remove 2 items
Replace Items Using
splice()
The splice() method is not just for removing or inserting — it can also replace items:
let languages = ["JavaScript", "Python", "C++"];
languages.splice(1, 1, "TypeScript");
// Replaces "Python" with "TypeScript"
🧠 A Note on Immutability
Many array operations modify the original list. If you need to preserve the original, use methods that return a new list (like slice, map, etc.), or copy the array using the spread operator:
let copy = [...languages];
Now that you’re comfortable working with items inside a JavaScript list, in the next section, we’ll look at commonly used methods that make array handling even more powerful and expressive.
Conclusion
Understanding how to work with JavaScript lists — or arrays, as they are officially called — is fundamental for any JavaScript developer. Though the term list is often used informally, it is arrays that provide the ordered, flexible data structure needed to store and manipulate collections of data.
In this post, we explored what a JavaScript list really is, how it differs from lists in other languages, and various ways to create, access, modify, and loop through these arrays. We also looked at some of the most useful built-in methods that simplify everyday tasks, from adding and removing elements to transforming and filtering data.