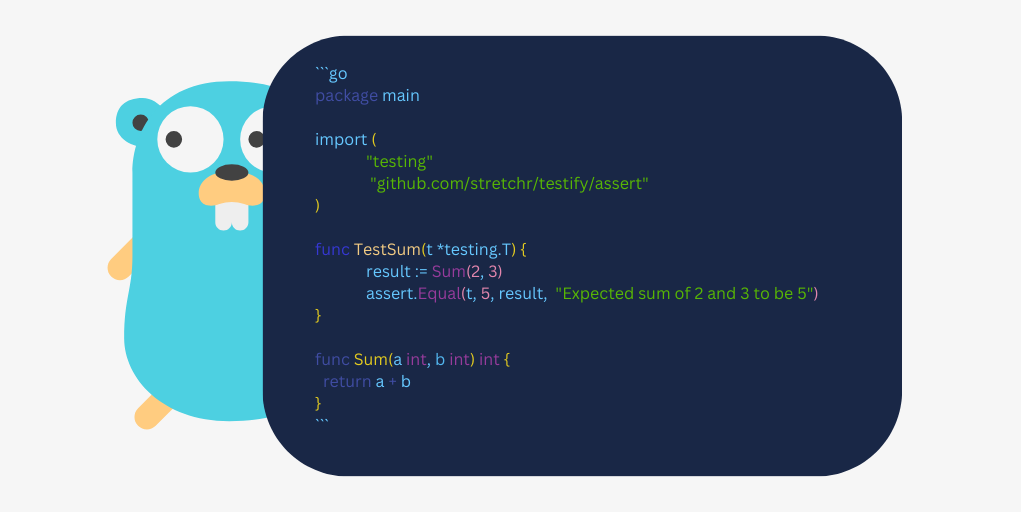
Golang Testing
Testing in Golang with Examples
Introduction Testing is an essential part of software development that ensures the reliability and quality of your code. In Go (Golang), testing is built into the language with a rich set of tools and features. This blog post will guide you through testing in Golang with practical examples and best