Understanding Relationships in MongoDB Database
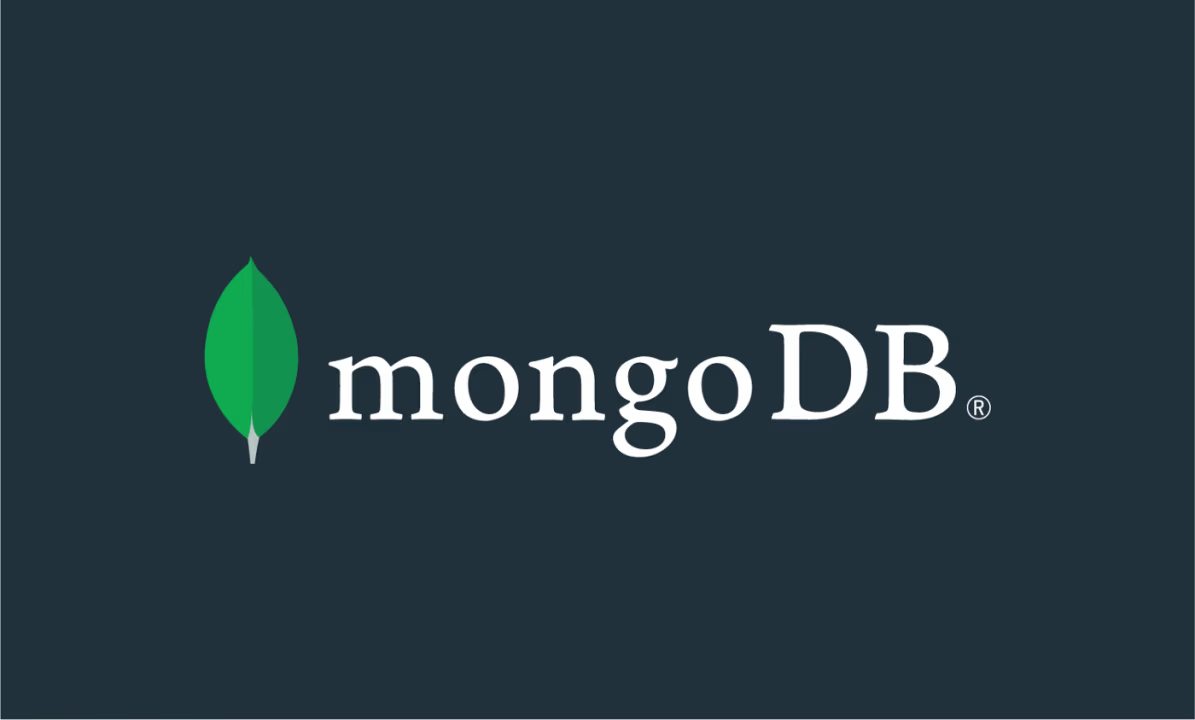
In the world of databases, understanding the nuances of how relationships are structured and managed is critical for developing scalable and efficient applications. MongoDB, a leading NoSQL database, provides a flexible model for handling relationships between data. Given its schema-less design, developers have unique methodologies to represent and fetch related data. This article delves into the intricacies of managing relationships in MongoDB, shedding light on optimal practices for leveraging relationships within this powerful database system.
Introduction to MongoDB
MongoDB is renowned for its document-based architecture which is built to handle large amounts of data across distributed systems. Unlike traditional relational databases, MongoDB stores data in flexible, JSON-like documents within collections, allowing for more dynamic schemas. This document model aligns closely with how developers typically write code, making it intuitive to use and easy to scale. However, handling complex relationships in database MongoDB environments can be a little different from what is done in relational databases.
Nature of Relationships in MongoDB
In traditional relational database management systems (RDBMS), relationships are achieved via joins that link data across tables using primary and foreign keys. MongoDB, on the other hand, offers two primary methods for representing relationships: embedded documents and references. These methodologies cater to different use cases, offering flexibility in how one can structure data.
1. Embedded Documents
One of the simplest ways to manage relationships in MongoDB is by utilizing embedded documents. This approach is generally used when one entity’s data is self-contained within another entity.
Use Case: Suppose you have a blog application where each post contains comments. Since comments are tightly related and are typically queried alongside their respective posts, embedding them within the post documents can be advantageous.
Advantages:
- Atomic Updates: Since both the post and its comments are in a single document, updates can be carried out atomically.
- Fewer Joins: Retrieval is efficient since related data is accessed within a single query.
- Simplicity: The schema design is straightforward, saving time during development.
Limitations:
- Document Size Limit: MongoDB documents have a size limit of 16 MB, thus embroiling a large set of embedded documents can breach this limit.
- Duplication Across Collections: In cases where documents need to be accessed separately or shared across collections, this approach may result in redundant data duplication.
2. Referencing
Referencing, through document linking via ObjectIds, comes into play when entities are sufficiently independent or when data integrity and reusability are paramount.
Use Case: For an e-commerce application, products and customer orders could be managed more effectively using references, allowing the product information to persist independently across different orders.
Advantages:
- Data Integrity: Changes in data are automatically reflected across all documents referencing it.
- Efficiency for Large Collections: Reduces redundancy when dealing with large repeating data sets.
Limitations:
- Complex Joins: To retrieve referenced data, additional queries are needed, which can result in complex aggregation queries or multiple database operations.
- Performance Implications: Fetching related documents via multiple queries can decrease read performance.
Implementing Relationships with MongoDB
To illustrate the above concepts, let’s explore some practical MongoDB implementations using both embedded documents and references.
Embedded Documents Example
Consider a simple e-commerce application where each order includes customer details, products purchased, and order status. An embedded document structure for the orders collection could look like this:
{
"_id": "order_id",
"customer": {
"name": "John Doe",
"email": "john.doe@example.com"
},
"items": [
{
"productId": "product_id_1",
"quantity": 2,
"price": 100
},
{
"productId": "product_id_2",
"quantity": 1,
"price": 200
}
],
"status": "shipped"
}
References Example
For a more complex scenario, where products are managed independently and reusable across various orders, here’s how reflecting relationships in MongoDB using references might look:
Products Collection:
{
"_id": "product_id",
"name": "Smartphone",
"price": 500,
"brand": "BrandX"
}
Orders Collection:
{
"_id": "order_id",
"customerId": "customer_id",
"items": [
{ "productId": "product_id", "quantity": 1 }
],
"status": "processing"
}
For fetching such related data with references, you might use MongoDB’s aggregation framework or incorporate additional driver logic in your application.
Aggregation Framework and the $lookup Operator
MongoDB utilizes the $lookup
operator within its aggregation framework to simulate joins between collections. This provides a powerful approach to querying referenced documents.
For instance, joining the orders and products collection to fetch complete order details might involve:
db.orders.aggregate([
{
$lookup: {
from: "products",
localField: "items.productId",
foreignField: "_id",
as: "productDetails"
}
},
{ $unwind: "$items" },
{ $match: { "items.productId": "desired_product_id" } }
])
This query highlights the versatility of MongoDB’s aggregation capabilities in fetching related data while still respecting MongoDB’s design philosophy.
Decision-Making for Relationships in MongoDB
When choosing between embedded documents and referencing within MongoDB, consider the following factors:
- Access Patterns: If your application frequently accesses multiple related entities independently, referencing might be preferred.
- Write vs. Read Operations: Prioritize embedding for read-heavy operations where joined data is needed, and references for write-heavy operations requiring frequent updates.
- Data Integrity and Consistency: For data that needs consistent updates across the dataset, lean towards referencing.
- Growth of Data: Predict the scalability needs and consider embedding for smaller sets and referencing for larger, evolving datasets.
Conclusion
Handling relationships in database MongoDB environments requires a strategic approach, balancing between embedding and referencing methodologies. By understanding the underlying principles and evaluating the specific needs of your application, you can effectively manage relationships, ensuring both performance and scalability. As MongoDB continues to evolve, keeping abreast of best practices will empower developers to harness the full potential of this dynamic database technology. Whether you are building simple applications or architecting large-scale distributed systems, the right choice of relationship management can significantly impact your application’s performance and maintainability.