Understanding The Null and Undefined in JavaScript
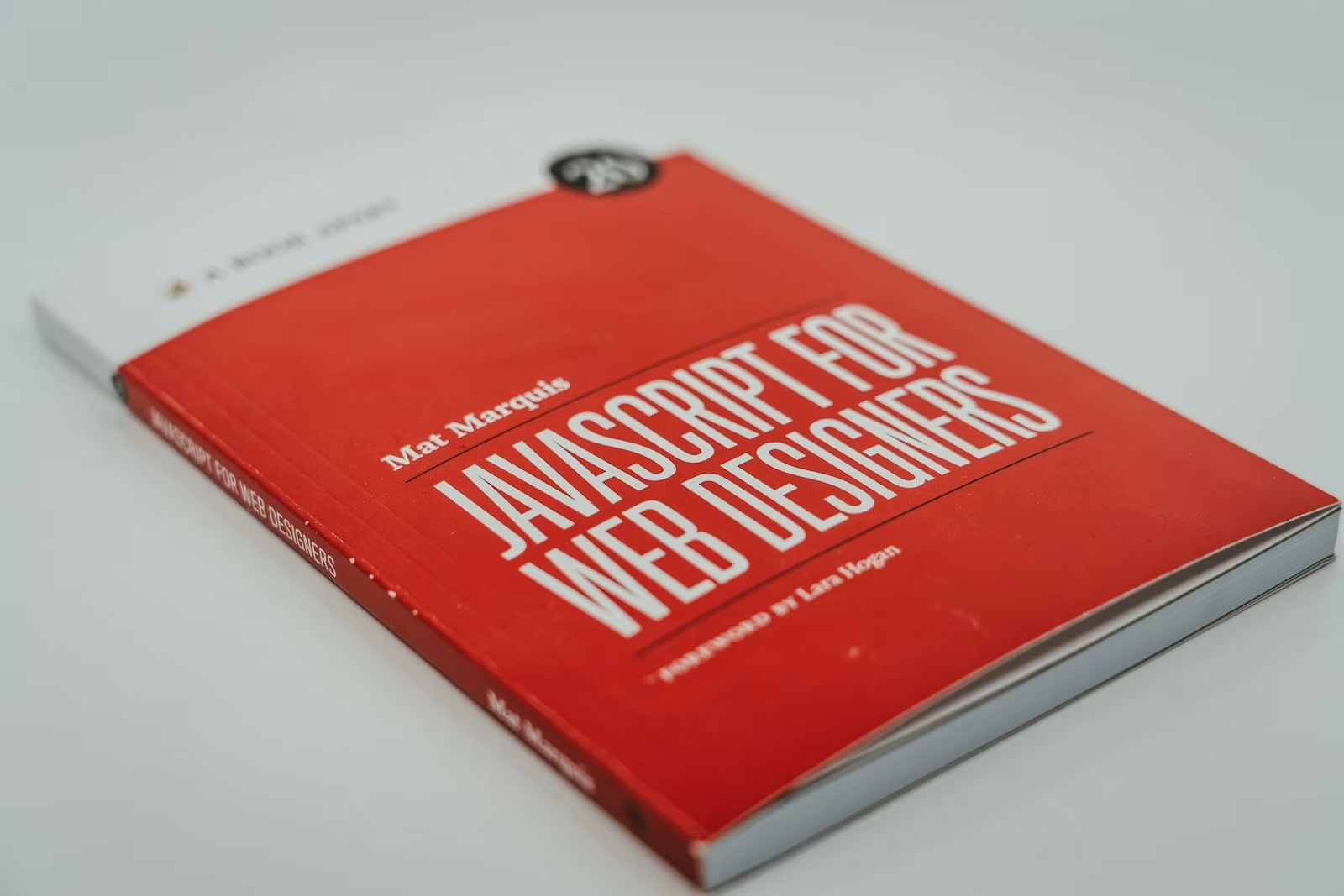
JavaScript, as a dynamic, loosely typed programming language, presents unique challenges and features, especially when it comes to handling data types. Among the myriad data types and constructs, two of the most commonly misunderstood and misused values are null
and undefined
. Understanding the difference between null and undefined in JavaScript is critical for developers aiming to write efficient and bug-free code. Although they might seem similar at first glance, these two types serve different purposes and can lead to different outcomes when incorporated into your programming logic.
What are null
and undefined
?
Before delving into the differences, it’s important to have a clear grasp of what these two values represent.
What is undefined
?
In JavaScript, undefined
is a primitive type that indicates the absence of a value. It’s what you get when you:
- Declare a variable without assigning it a value:
let a;
console.log(a); // Outputs: undefined
- Call a function without a return statement and then inspect the function’s return value:
function doSomething() {}
console.log(doSomething()); // Outputs: undefined
- Access an object property that doesn’t exist:
const obj = {};
console.log(obj.nonExistentProperty); // Outputs: undefined
What is null
?
On the other hand, null
is an assignment value that can be used to represent “no value.” It explicitly expresses the absence of any object value or the intentional non-existence of any object that a variable could point to.
- Explicitly assign
null
to signify “no value” or “empty object”:
let user = null;
- Use
null
to indicate an empty object or to reset a variable:
let data = { key: 'value' };
data = null; // data no longer holds any object reference
Key Differences Between null
and undefined
Understanding the difference between null and undefined in JavaScript involves uncovering the nuanced roles they play within the language:
1. Data Type
null
: In JavaScript,typeof null
returns an object. This is a well-known bug in JavaScript that originates from its early implementations. Despite this quirk,null
is generally regarded as a distinct primitive type.
console.log(typeof null); // Outputs: object
undefined
: Consistently a primitive type;typeof undefined
returns “undefined.”
console.log(typeof undefined); // Outputs: undefined
2. Usage
- Initialization vs. Assignment:
undefined
is typically the default state of variables when they are declared but not initialized. In contrast,null
is assigned by developers to indicate that a variable should be intentionally empty. - Control Flow: Using
null
in control flow (checks and conditionals) can signal a deliberate state for variables, which may help simplify debugging and flow control logic.
3. Best Practices
- Avoiding Unexpected Errors: Assign
null
to variables when you want to explicitly indicate that they are empty, rather than leaving them uninitialized. This practice avoids accidental errors related to accessing or operating on uninitialized variables. - Function Arguments and Return Values: For function parameters that may legitimately have no value, it’s often safer and more expressive to use
null
rather than letting them default toundefined
.
4. Comparison
- Loose Equality (
==
): In JavaScript, comparingnull
andundefined
with double equals (==
) returns true because non-strict equality checks convert both values to a common type (in this case,null
).
console.log(null == undefined); // Outputs: true
- Strict Equality (
===
): Strict equality (===
) operates without type conversion, thereby distinguishing betweennull
andundefined
.
console.log(null === undefined); // Outputs: false
5. JSON Serialization
- JSON.stringify: When serializing objects into JSON format,
undefined
properties are omitted entirely, whilenull
properties are retained.
let obj = { a: undefined, b: null };
console.log(JSON.stringify(obj)); // Outputs: {"b":null}
6. Considerations in Function Calls
- Function Parameters: When you call a function with missing arguments, those absent arguments are implicitly assigned
undefined
. Conversely, setting a function argument tonull
explicitly communicates a meaningful lack of value.
Common Pitfalls and How to Avoid Them
JavaScript’s flexibility with null
and undefined
can sometimes lead to common pitfalls that developers must guard against.
Mistake 1: Forgetting to Initialize Variables
As mentioned previously, failing to initialize a variable results in undefined
, which could lead to unexpected behavior when performing operations that expect a defined value. Always initialize variables, preferably with null
if their values cannot be determined immediately.
Mistake 2: Ignoring Type Coercion
Type coercion can cause logic errors within code when improperly comparing null
and undefined
. Developers should employ strict equality operators (===
) to circumvent unintended type conversion.
Mistake 3: Misunderstanding Default Behavior
Recall that function return values default to undefined
. If developers mistakenly assume all function calls yield meaningful outputs, they might inadvertently operate with undefined
, causing further issues downstream.
Mistake 4: Inadvertent Object Usage
Attempting to interact with null
or undefined
as if they were objects will throw type errors. Developers should proactively validate these variables before including them in object operations or property accesses.
let item = null;
// Guard clause to prevent TypeError
if (item) {
// Perform operations with 'item'
}
Conclusion
Grasping the difference between null and undefined in JavaScript is foundational for developers aiming to write powerful and maintainable code. Each has a specific context and purpose that, when used correctly, can greatly enhance clarity and functionality within applications. Rather than simply accepting these constructs as interchangeable, developers should engage with them intentionally, striving for code that speaks directly to user needs and business requirements.
By focusing on explicit initialization, embracing strict comparison, acknowledging JSON serialization behaviors, and anticipating error conditions, you can wield these tools to elevate your JavaScript development rigor. Through a deeper understanding of null
and undefined
, you’ll be better equipped to troubleshoot issues, write cleaner code, and build more robust applications.