How Does Golang Handle Time and Duration?
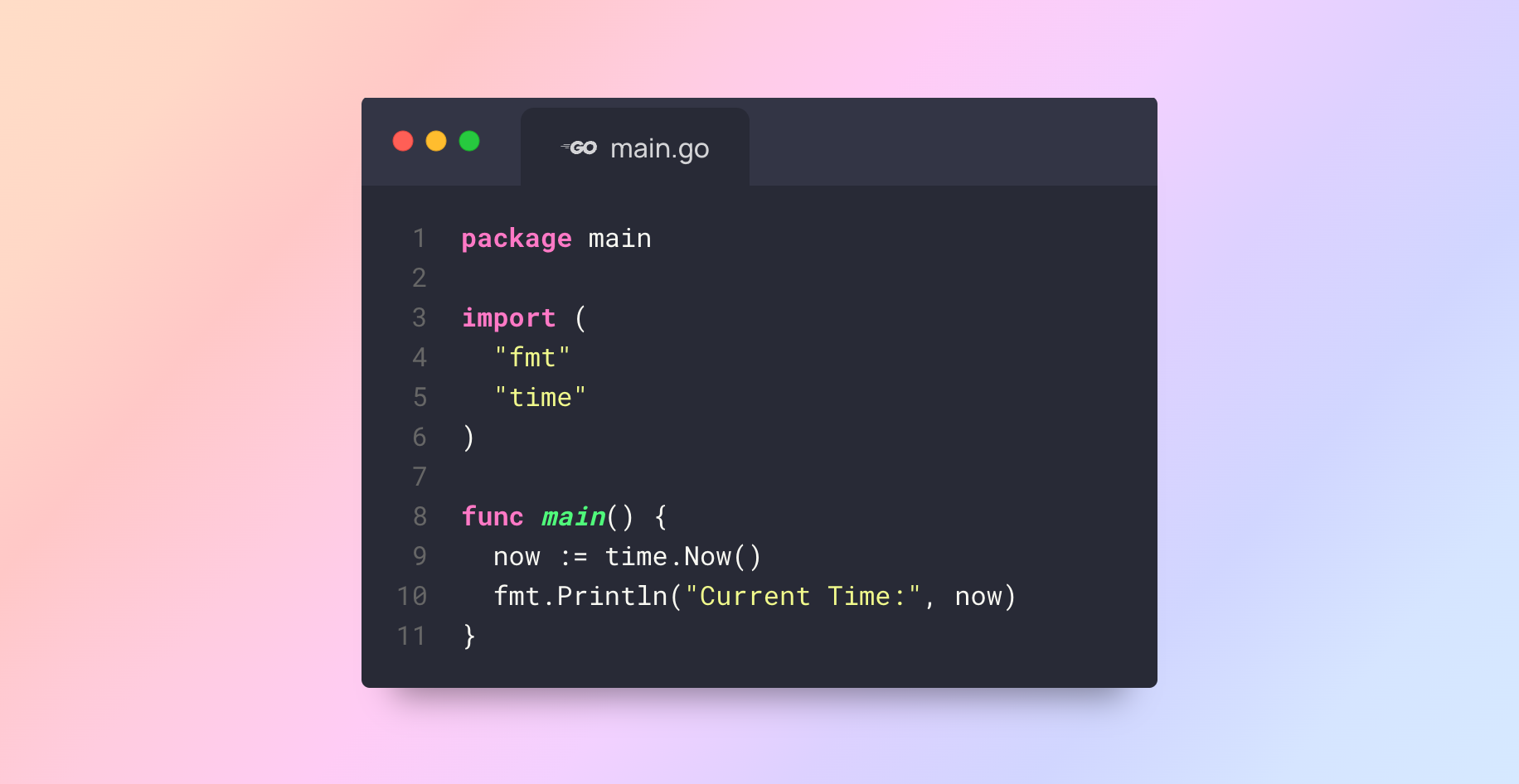
Working with time in Go is pretty straightforward once you get the hang of it. Go’s standard library provides a powerful time
package that lets you do everything—from checking the current time, calculating durations, formatting dates, to even parsing strings into time.
In this article, we’ll go through:
- Getting the current time
- Working with
Duration
- Adding and subtracting time
- Formatting and parsing time
- Sleeping and timers
1. Getting the Current Time
To get the current time in Go, you can use time.Now()
.
package main
import (
"fmt"
"time"
)
func main() {
now := time.Now()
fmt.Println("Current Time:", now)
}
Sample Output:
Current Time: 2025-04-09 13:45:00.123456789 +0530 IST
You can get parts of the time using methods like:
fmt.Println("Year:", now.Year())
fmt.Println("Month:", now.Month())
fmt.Println("Day:", now.Day())
fmt.Println("Hour:", now.Hour())
2. What is time.Duration
?
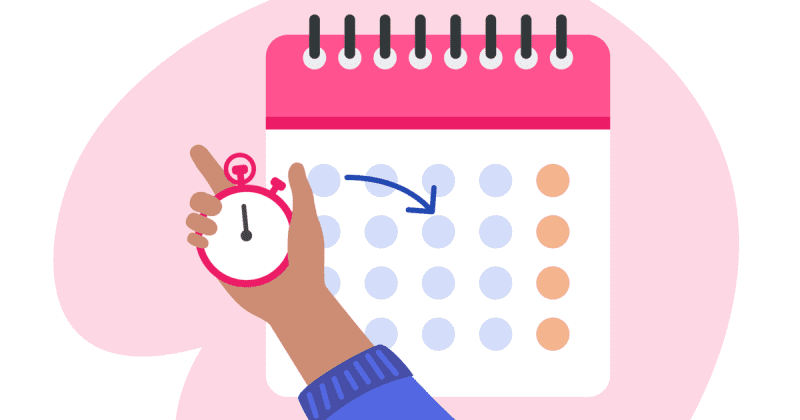
time.Duration
represents the difference between two time.Time
values. It’s internally stored as nanoseconds, but we usually use helpers like time.Second
, time.Minute
, etc.
duration := 2 * time.Hour
fmt.Println("Duration in hours:", duration.Hours())
You can create custom durations like:
myDuration := time.Duration(90) * time.Minute
fmt.Println("My Duration:", myDuration)
3. Adding and Subtracting Time
Working with time is a common task in any application—whether you’re scheduling tasks, setting timeouts, or calculating durations. Thankfully, Go’s standard time package makes it super easy to add or subtract time, and even compare two time values. In this quick guide, we’ll look at how to perform time arithmetic in Go using simple, real-world examples.
Add 1 hour and 30 minutes:
future := time.Now().Add(1*time.Hour + 30*time.Minute)
fmt.Println("Future Time:", future)
Subtract 1 day:
past := time.Now().Add(-24 * time.Hour)
fmt.Println("Yesterday:", past)
Time difference:
t1 := time.Now()
t2 := t1.Add(2*time.Hour + 10*time.Minute)
diff := t2.Sub(t1)
fmt.Println("Difference:", diff)
4. Formatting Time in Go
Formatting dates and times in Go is a bit different compared to other languages. Instead of using format strings like "YYYY-MM-DD", Go uses a unique reference date: Mon Jan 2 15:04:05 MST 2006. It might look weird at first, but once you get the hang of it, formatting time in Go becomes pretty easy and powerful. In this guide, we’ll walk through how to format and display time values in various styles using Go’s time package.
Go uses a weird but fixed layout to format time:
"Mon Jan 2 15:04:05 MST 2006"
Yes, that’s the actual reference time.
now := time.Now()
formatted := now.Format("2006-01-02 15:04:05")
fmt.Println("Formatted Time:", formatted)
More examples:
fmt.Println("Date Only:", now.Format("02 Jan 2006"))
fmt.Println("Time Only:", now.Format("03:04 PM"))
5. Parsing Strings into Time
Sometimes you get a date or time as a string—maybe from user input, an API, or a config file—and you need to convert it into a proper time.Time value in Go. This process is called parsing, and in Go, it’s done using the time.Parse() function. Just like formatting, Go uses its unique reference time layout (Jan 2 2006 15:04:05) to understand how your string is structured. In this section, we’ll look at how to parse date/time strings into usable time.Time objects with practical examples.
To convert a string into time.Time
:
layout := "2006-01-02 15:04:05"
str := "2025-04-09 10:30:00"
parsedTime, err := time.Parse(layout, str)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Parsed Time:", parsedTime)
}
6. Sleep and Timers
In Go, if you want to pause your program for a while or run something after a delay, you’ll be working with time.Sleep and time.Timer. These are handy tools for scheduling, throttling, and adding delays without blocking the entire program. In this section, we’ll look at how to pause execution for a set duration, and how to use timers to trigger actions after a specific time.
Pause execution:
fmt.Println("Sleeping for 2 seconds...")
time.Sleep(2 * time.Second)
fmt.Println("Awake!")
Using time.After
:
ch := time.After(3 * time.Second)
fmt.Println("Waiting...")
<-ch
fmt.Println("3 seconds passed.")
7. Tickers for Repeated Tasks
If you need to run a task repeatedly at fixed intervals—like checking a server’s health, syncing data, or printing logs—Go’s time.Ticker is your friend. A ticker sends a signal on a channel at regular intervals, letting you run code on a loop without manually managing the timing. In this section, we’ll see how to use tickers for scheduling repeated tasks, and how to stop them cleanly when you’re done.
Tickers run something on intervals:
ticker := time.NewTicker(1 * time.Second)
defer ticker.Stop()
for i := 0; i < 5; i++ {
fmt.Println("Tick at", <-ticker.C)
}
Final Thoughts
The time
package in Go handles most real-world use-cases like:
- Scheduling tasks
- Measuring time between events
- Formatting logs
- Sleep and timers
🔖 Quick Cheat Sheet
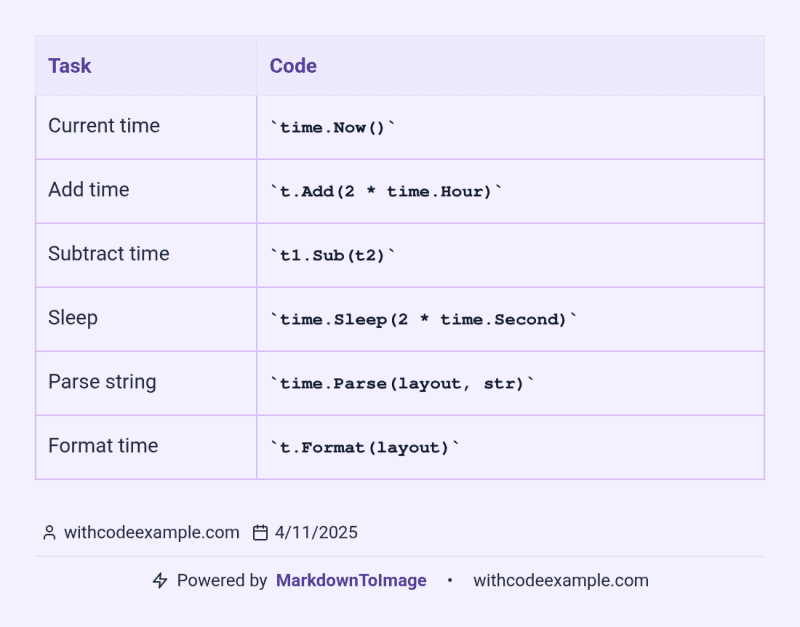
Conclusion
Time handling in Go might look a bit quirky at first—especially the formatting part—but once you get used to it, it’s super clean and predictable. Whether you’re building a scheduler, doing time-based logging, or writing an uptime monitor, the time package gives you all the necessary tools without overcomplicating things. Just keep the reference layout in mind and you’re good to go.
That’s it! Hope this helped clear up how time and duration work in Go. If you’ve got questions or want to see more examples, feel free to ask in the comments. Happy coding! 🧑💻⌛