A Comprehensive Guide to Installing Packages Using Go Get
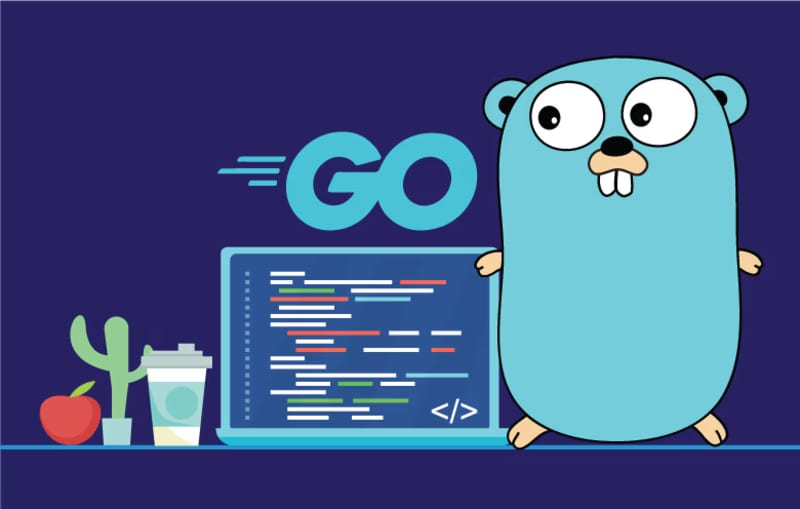
Go, also known as Golang, is a statically typed, compiled programming language designed by Google. It is famous for its simplicity, performance, and concurrent capabilities. One of the many reasons developers prefer Go is its efficient package management system. This guide will focus on understanding how to install a package using the command go get
, an integral tool for Go developers that simplifies managing dependencies in Go projects.
What is Go Get?
Before diving into the installation process, let’s understand the basics of the go get
command. When you’re developing a Go application, it’s essential to utilize existing code libraries or packages available in the Go ecosystem. go get
is a built-in Go command that allows you to fetch, compile, and install packages from remote repositories, which are primarily hosted on platforms like GitHub, Bitbucket, or custom VCS (Version Control System) repositories.
Why Use Go Get?
The power of go get
lies in its capability to handle dependencies automatically, ensuring that your Go workspace contains all the packages your application requires. Here’s why you should use go get
:
- Simplifying Dependency Management: Automatically downloads and installs the package along with all its dependencies.
- Ease of Use: Minimal effort required to integrate third-party libraries into your projects.
- Versatile: Works with various version control systems and can fetch packages from private or public repositories.
- Maintaining Up-to-date Packages: Easily update packages to their latest versions.
How Can I Install a Package with Go Get?
To address the important question — “How can I install a package with go get?” — follow the comprehensive steps below for an efficient installation process. We will also highlight common use-cases and best practices to enhance your understanding and efficiency with go get
.
Step-by-step Installation Guide
1. Setting Up Your Go Environment
Before installing any package, ensure your Go environment is correctly set up:
- Install Go: Download the latest version of Go from the official Go website.
- Set Go Path: Although with Go Modules, setting
$GOPATH
is less critical; it’s still vital for legacy projects. Usego env
to check your current environment settings and$GOPATH/bin
to your$PATH
for executing installed binaries.
Ensure that your workspace is ready by verifying the installation with:
$ go version
2. Initializing a Go Module
With the introduction of Go Modules, you no longer need to depend entirely on GOPATH. To get started with Go Modules, create or navigate to your project directory and initialize a Go module:
$ mkdir my_project
$ cd my_project
$ go mod init my_project
This command creates a go.mod
file, which serves as a module definition file, capturing the exact versions of all dependencies your project requires.
3. Installing a Package
To install a package, use the go get
command followed by the package import path. For example, to install the popular HTTP router github.com/gorilla/mux
:
$ go get -u github.com/gorilla/mux
- Flag Explanation:
- The
-u
flag updates the package and its dependencies.
The above command downloads the package and enables your project to use it, creating or updating the go.mod
and go.sum
files to lock the dependencies required.
4. Importing the Package in Your Code
Once a package is installed, the next step is to import it into your Go application:
package main
import (
"fmt"
"github.com/gorilla/mux"
)
func main() {
r := mux.NewRouter()
fmt.Println("Router created:", r)
}
5. Removing a Package
To remove a dependency, simply remove any reference to it in your code and run:
$ go mod tidy
This removes unused dependencies from go.mod
and go.sum
.
Understanding Go Get with Modules
With the introduction of Go Modules, go get
has evolved significantly:
- Working Outside GOPATH: Modules allow working outside the traditional GOPATH structure, simplifying the integration of Go codebases.
- Versioning: Dependencies can be pegged to specific versions, ensuring consistent behavior across different environments.
- Proxy Support: Improves package fetch reliability, as Go can fetch modules via a proxy.
Common Issues and Troubleshooting
Occasionally, you might encounter issues while installing packages with go get
. Here are a few debugging tips:
- Network Issues: Package installation may fail due to network connectivity problems. Ensure network access to the repository and try again.
- Permission Issues: Ensure you have the necessary permissions for the directory where Go installs its packages.
- Module Path Conflicts: If there’s a conflict in the module path, ensure the correct module path is set and update your
go.mod
file accordingly.
Best Practices
- Use Modules Over GOPATH: Prefer modules for a modern Go workflow, allowing for reliable package management outside of GOPATH.
- Regularly Update Packages: Schedule regular updates for dependencies to benefit from improvements and security patches.
- Version Control: Pin versions of your dependencies explicitly to avoid unexpected errors due to upstream changes.
Conclusion
The go get
command is an essential tool in the Go ecosystem, providing a streamlined process for managing dependencies. By following this guide, you now have a comprehensive understanding of how to install a package with go get
, leveraging Go Modules for efficient and manageable package handling. As you continue your journey in Go development, keep these practices and steps in mind to maintain a robust and reliable codebase. Happy coding!