Mastering Golang: A Comprehensive Guide to Go Programming
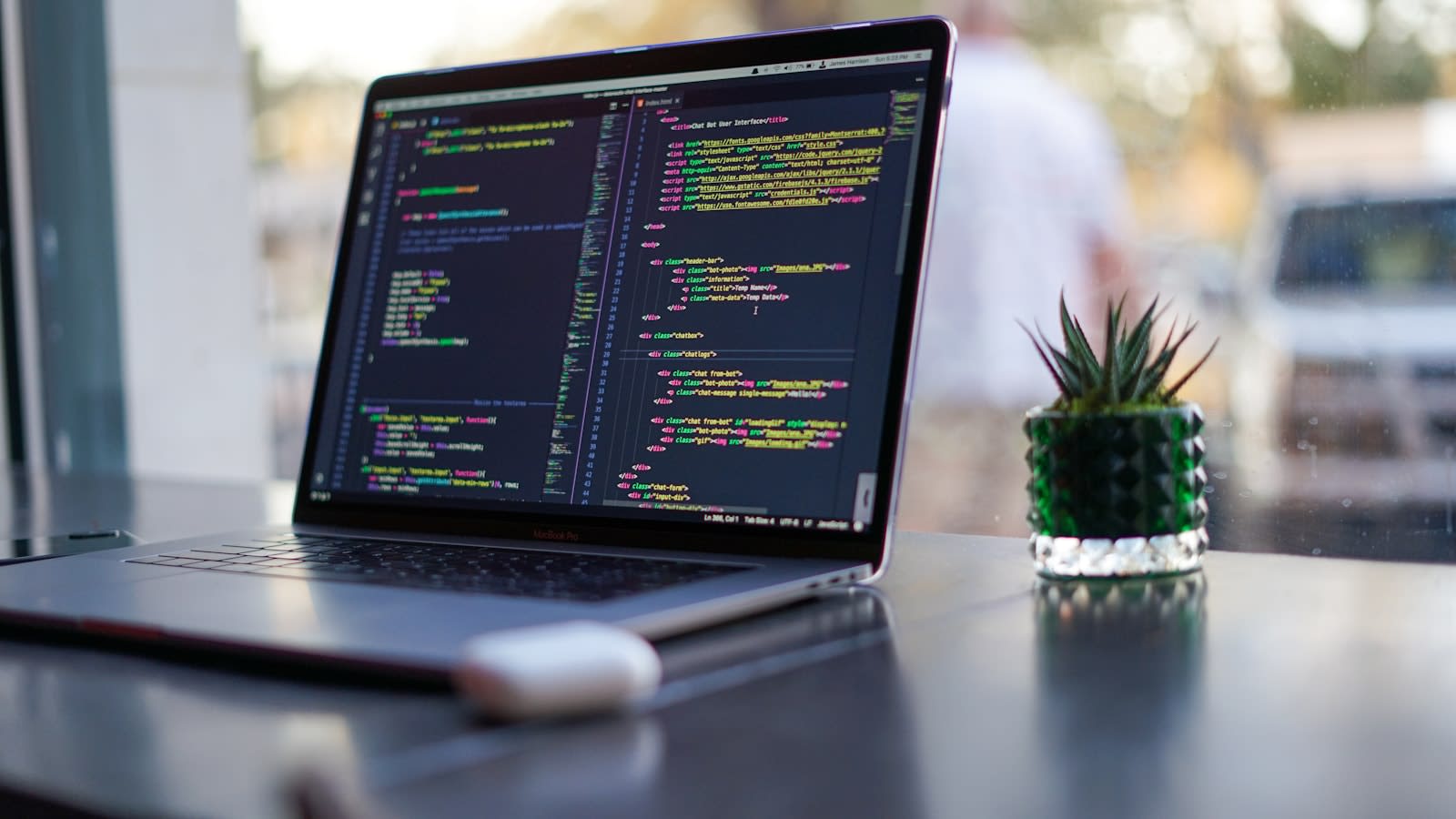
The world of programming languages is vast and varied, with each language offering its own unique set of features and benefits. Among these, Golang, also known as Go, stands out for its efficiency, simplicity, and robustness. Since its inception, Go has garnered a substantial following among developers for its ability to simplify complex processes and improve performance. In this comprehensive guide, we delve into the core aspects of Golang programming, helping you master this powerful language and optimize your projects effectively.
Introduction to Golang
Golang was developed by Google engineers Robert Griesemer, Rob Pike, and Ken Thompson, and released to the public in 2009. It was designed to address some of the shortcomings of languages they were using, such as C++ and Java, by providing a fast, easy-to-use, and statically typed language that supports concurrent programming.
Unlike many contemporary languages, Golang offers a simplified syntax similar to C, making it straightforward for beginners while providing the power needed by seasoned developers. Its efficiency in memory management, along with features like garbage collection and dependency management, makes Golang ideal for building scalable, high-performance applications.
The Core Features of Golang
1. Simplicity and Readability
One of Golang’s biggest strengths is its simplicity. The language was intentionally designed to limit complexity and increase readability, making it easier to write and understand code. Its clean syntax resembles C, but eliminates many of the nuanced intricacies found in other languages, such as C++.
2. Concurrency Model
Concurrency is a primary focus in Golang. The language provides built-in support for concurrent programming, enabling developers to efficiently manage multiple tasks simultaneously. Go’s concurrency model is based on goroutines and channels, which allows for efficient communication between threads without the heavy-handedness of traditional threading models.
3. Fast Compilation
Golang is known for its fast compilation times, which greatly enhances developer productivity. The compilation process is swift because Go works with a single binary. This feature allows large projects to compile quickly, a notable benefit for developers accustomed to the slower compilation times of languages like Java.
4. Robust Standard Library
Golang boasts a powerful and extensive standard library that includes numerous packages for handling tasks such as I/O, networking, cryptography, and web servers. This library empowers developers to build applications more efficiently, reducing the need for countless third-party dependencies.
5. Static Typing and Garbage Collection
Being a statically typed language, Golang checks types at compile time, reducing runtime errors. At the same time, Go provides automatic memory management through garbage collection, akin to languages like Java. This feature minimizes the programmer’s burden of memory allocation and deallocation.
Setting Up Golang Environment
Installing Go
Before diving into Golang programming, the first step is to set up the Go environment on your system. You can download the latest version of Go from its official website. The installation process is relatively straightforward as Go provides binaries for several platforms, including Windows, macOS, and Linux.
Setting Up Your Go Workspace
After installation, setting up a Go workspace is crucial. A well-structured workspace typically consists of three main directories: src
, pkg
, and bin
. The src
directory contains source files, pkg
holds compiled files, and bin
stores executable files. Configuring the Go workspace correctly ensures that your projects have the necessary structure to support scalable development.
Configuring Environment Variables
To effectively work with Go, you need to set the GOPATH
environment variable, which points to your workspace directory. Additionally, adding Go’s binary directory to your PATH
ensures you can execute Go commands from any location in your terminal.
Writing Your First Go Program
With the environment set up, let’s write a simple Go program. Open your text editor and create a new file named hello.go
in the src
directory.
package main
import "fmt"
func main() {
fmt.Println("Hello, Golang!")
}
This basic program introduces you to the main
package, the import
statement for including the fmt
package, and the main
function, which is the entry point for every Go program. Save the file and run it using the Go command:
go run hello.go
If everything is set up correctly, you should see the output “Hello, Golang!” in your terminal.
Delving Deeper into Golang Concepts
Functions and Packages
Functions in Golang are defined using the func
keyword, followed by a function name and parameters. Here’s a simple example of a function that adds two integers:
func add(a int, b int) int {
return a + b
}
Golang’s use of packages is a core feature that promotes organized code structure. Every Go program is made up of packages, and by organizing code into packages, you can easily manage complex applications with multiple components.
Structs and Interfaces
Golang does not support classes in the traditional sense but offers structs and interfaces as alternatives. Structs are collections of fields, while interfaces are types that specify method signatures.
type Rectangle struct {
width float64
height float64
}
func (r Rectangle) Area() float64 {
return r.width * r.height
}
In this example, the Rectangle
struct represents geometric shapes, and an associated method Area
calculates its area.
Error Handling
Error handling in Golang is explicit and straightforward. Functions that might encounter an error return an additional return value to indicate the error.
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, fmt.Errorf("cannot divide by zero")
}
return a / b, nil
}
Goroutines and Channels
Goroutines are a lightweight alternative to threads, allowing concurrent execution of functions. Channels facilitate communication between goroutines, enabling them to synchronize their tasks without shared memory.
func printNumbers() {
for i := 1; i <= 5; i++ {
fmt.Println(i)
}
}
func main() {
go printNumbers()
fmt.Println("Numbers printed concurrently")
}
In this code snippet, printNumbers
runs concurrently with other operations, demonstrating Go’s elegant approach to managing concurrency.
Conclusion
Mastering Golang involves understanding its simplified syntax, powerful libraries, and unique concurrency model. Whether you’re building web applications, cloud computing solutions, or microservices, Golang’s efficiency and performance make it a compelling choice for developers. As you deepen your knowledge of Golang, you will find that its simplicity and power enable you to create robust, high-performing applications with ease.
By following this comprehensive guide, you can accelerate your journey to becoming proficient in Golang programming, harnessing its full potential in your software development endeavors. As you continue your exploration of this versatile language, keep experimenting with new features, contributing to Go’s vibrant open-source community, and staying updated with its latest developments.