Testing in Golang with Examples
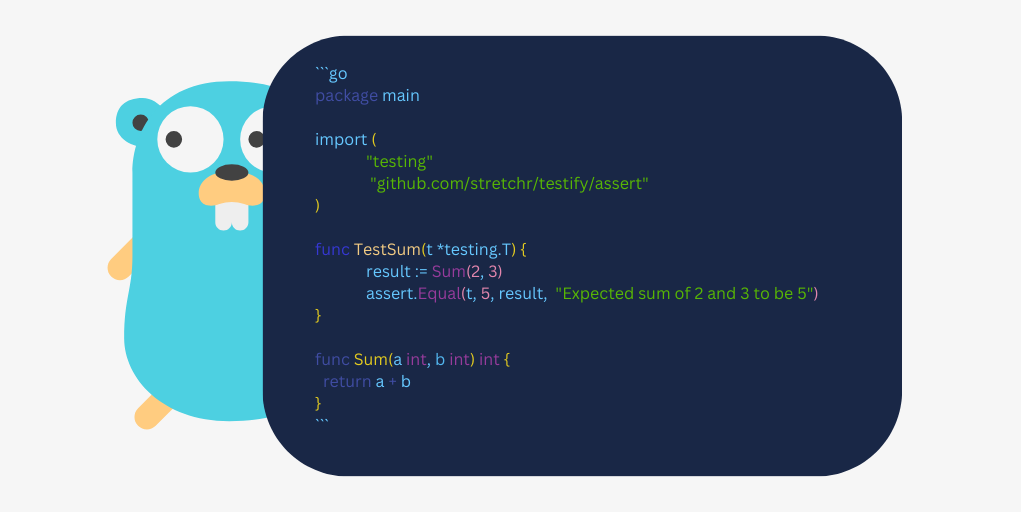
Introduction
Testing is an essential part of software development that ensures the reliability and quality of your code. In Go (Golang), testing is built into the language with a rich set of tools and features. This blog post will guide you through testing in Golang with practical examples and best practices.
1. Why Testing is Important in Golang
Testing helps identify bugs early in the development cycle, reduces the cost of fixing issues, and improves code quality. In Golang, testing can be more effective due to its emphasis on simplicity and maintainability. By integrating testing into your development workflow, you can:
- Ensure code correctness.
- Facilitate code refactoring.
- Provide documentation for your codebase.
- Enhance collaboration within teams.
2. Setting Up Your Go Environment for Testing
Before diving into writing tests, you need to ensure that your Go environment is properly set up. Follow these steps:
- Install Golang from the official site.
- Set up your workspace typically in the
~/go
directory. - Create a simple Go project using
[go mod init](https://withcodeexample.com/go-mod-init-dependency-management-go) <modulename>
.
Once your project is ready, you can create a*_test.go
file to hold your tests.
3. Writing Your First Test
In Go, testing functions follow a specific naming convention: they must start with the word Test
. Below is an example of a simple function and its corresponding test:
// main.go
package main
import "fmt"
func Add(a, b int) int {
return a + b
}
func main() {
fmt.Println(Add(3, 4))
}
// main_test.go
package main
import "testing"
func TestAdd(t *testing.T) {
result := Add(3, 5)
expected := 8
if result != expected {
t.Errorf("Add(3, 5) = %d; want %d", result, expected)
}
}
To run your tests, use the go test
command. This will automatically look for files ending with _test.go
and execute the test functions within them.
4. Understanding the Testing Package
The testing
package provides the tools you need to write and run tests. Here are some of the primary features:
- T: A struct type that carries test status and methods for logging.
- Benchmarking: Functions prefixed with
Benchmark
can be used to run performance tests. - Subtests: Allows organization of tests into groups for better clarity.
Example of Subtests:
func TestAdd(t *testing.T) {
tests := []struct {
a, b, expected int
}{
{1, 2, 3},
{2, 3, 5},
{3, 4, 7},
}
for _, test := range tests {
t.Run(fmt.Sprintf("%d+%d", test.a, test.b), func(t *testing.T) {
result := Add(test.a, test.b)
if result != test.expected {
t.Errorf("Add(%d, %d) = %d; want %d", test.a, test.b, result, test.expected)
}
})
}
}
5. Table-Driven Tests
Table-driven tests are a common pattern in Go that promotes clarity and maintainability. Instead of writing multiple test functions, you can create a table of test cases:
func TestAdd(t *testing.T) {
cases := []struct {
a, b, expected int
}{
{1, 2, 3},
{1, -1, 0},
{0, 0, 0},
{-2, -3, -5},
}
for _, c := range cases {
got := Add(c.a, c.b)
if got != c.expected {
t.Errorf("Add(%d, %d) = %d; want %d", c.a, c.b, got, c.expected)
}
}
}
This approach keeps your tests organized and makes it easy to add new test cases.
6. Writing Benchmarks
Go allows you to write benchmarks to measure the performance of your code. A benchmark function is defined similarly to a test function but starts with Benchmark
. Here is an example:
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(1, 1)
}
}
Run the benchmark using:
go test -bench=.
This will give you insight into how your functions perform under different loads.
7. Coverage Analysis
Go provides built-in tools to analyze test coverage, which indicates which parts of your code are being tested. To see coverage, run:
go test -cover
For more detailed coverage reports, you can use:
go test -coverprofile=coverage.out
go tool cover -html=coverage.out
This will generate an HTML report that visualizes the coverage of your codebase, helping you identify untested areas.
8. Best Practices for Testing in Golang
To write effective tests in Golang, consider these best practices:
- Keep tests isolated: Each test should not depend on external states.
- Use descriptive names: Clearly name your test functions to describe what they test.
- Leverage
t.Run
for subtests: Organize tests into subtests for better structure and output. - Test edge cases: Ensure you test not only typical use cases but also edge cases and error scenarios.
- Document tests: Explain non-obvious tests with comments to improve maintainability.
Conclusion
In summary, testing in Golang is straightforward and powerful, allowing developers to ensure code quality with minimal overhead. With the built-in testing
package, you can write unit tests, benchmarks, and coverage reports to maintain and improve your codebase. By adopting the practices outlined in this post, you will create a robust testing suite that fosters confidence in your Go applications.
Start testing your Golang projects today to experience the benefits firsthand!