Gin Framework Project Structure Tutorial Chapter 3 Guide
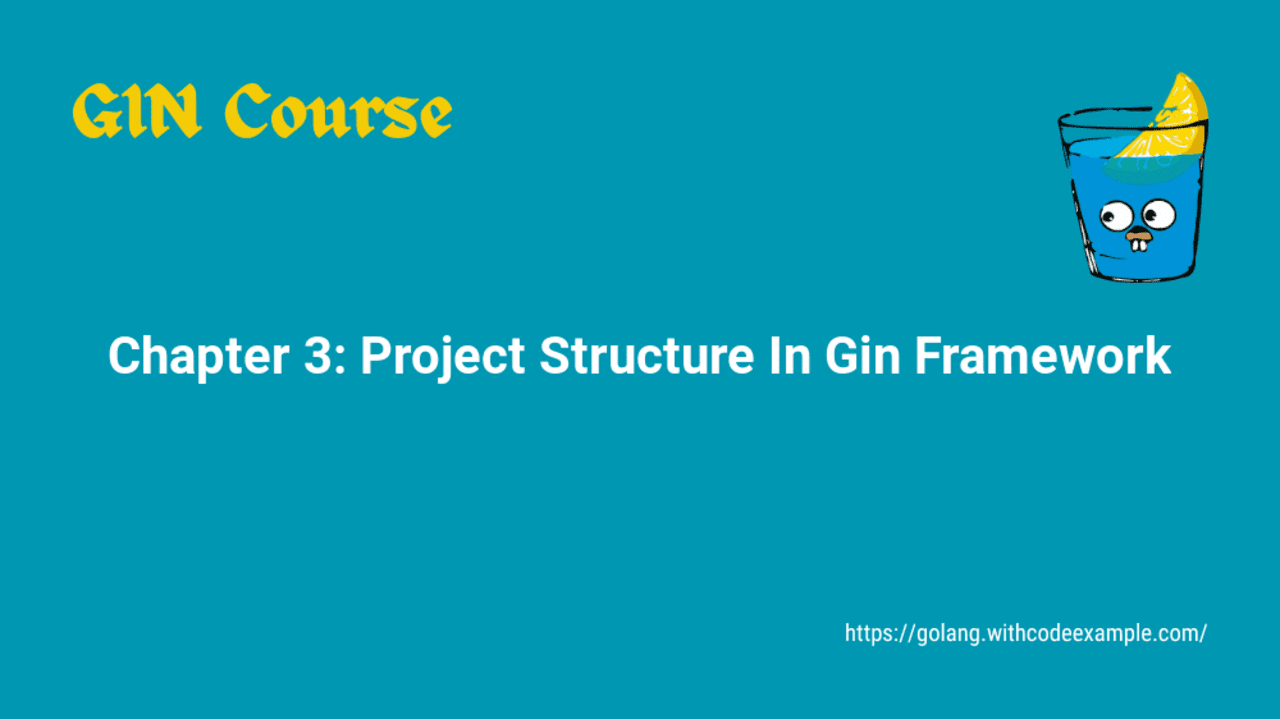
So, in chapter 3 of the Gin tutorial, we’re gonna talk about how to set up your project. It’s not just about throwing files around – it’s about making smart choices on where everything goes. Trust me, this stuff matters. If you do it right, your project will be way easier to work with down the road. You’ll thank yourself later when you’re not tearing your hair out trying to find stuff or add new features. Plus, it’ll make your code look way more pro. So let’s dig into the nitty-gritty of organizing your Gin project like a boss.
Prerequisites
Organizing Files and Directories
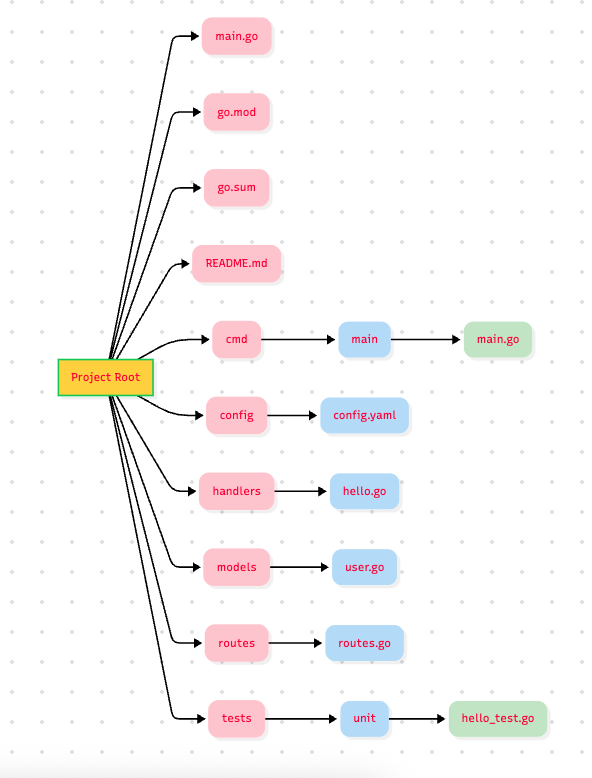
A well-organized project structure is fundamental for seamless development and collaboration. Here’s a recommended layout for a Gin project:
Main Directory:
main.go
: This file serves as the entry point of the application. It typically contains the initialization logic for your Gin router and other essential configurations.- go.mod and
go.sum
: These files are used for managing dependencies with Go modules. They list the project’s dependencies and their versions. README.md
: A README file containing essential information about the project, such as its purpose, installation instructions, and usage guidelines.
Internal Packages:
internal/
: This directory is reserved for packages that are specific to the project and not intended for use by external applications or libraries. It helps encapsulate project-specific logic and prevents unintended dependencies.pkg/
: Thepkg
directory houses reusable packages that may be shared across multiple projects. These packages should be well-documented and thoroughly tested to ensure their reliability and versatility.
Configuration:
config/
: This directory contains configuration files used to customize the behavior of the application. These files may include settings related to database connections, server ports, logging levels, and other runtime parameters.env/
: Theenv
directory typically stores environment-specific configuration files, such as development, staging, and production configurations. Separating configurations by environment helps maintain consistency and simplifies deployment workflows.
Handlers and Middleware:
handlers/
: Handlers are responsible for processing incoming requests and generating appropriate responses. Organize your handlers based on the routes they handle or the resources they manage to maintain clarity and coherence.middleware/
: Middleware functions intercept incoming requests before they reach the handlers, allowing you to perform common tasks such as authentication, logging, and request preprocessing. Separating middleware logic from route handlers promotes modularity and reusability.
Database Access:
repository/
: Therepository
directory contains database access and data retrieval logic. It encapsulates interactions with the database, including CRUD operations, transaction management, and query execution.models/
: Database models represent the structure of your data and define how it is stored and manipulated. Organize your models based on the entities they represent, such as users, products, or orders, to maintain clarity and consistency.
Utilities and Helpers:
utils/
: Utility functions encapsulate common tasks or operations that are used throughout the application. Examples include string manipulation, date formatting, and encryption utilities. Organize your utilities based on functionality to facilitate code discovery and reuse.helpers/
: Helper functions provide reusable snippets of code that assist in specific tasks or workflows. These functions are typically used to simplify complex operations or encapsulate common patterns. Keep your helper functions well-documented and modular to promote code readability and maintainability.
Static Assets and Templates:
static/
: Static assets such as CSS stylesheets, JavaScript files, and images are stored in thestatic
directory. These assets are served directly to clients by the web server and are typically used to enhance the presentation and functionality of web pages.templates/
: HTML templates define the structure and layout of dynamically generated web pages. By separating templates from code logic, you can maintain a clear separation of concerns and facilitate collaboration between front-end and back-end developers.
Configuring the Project Layout
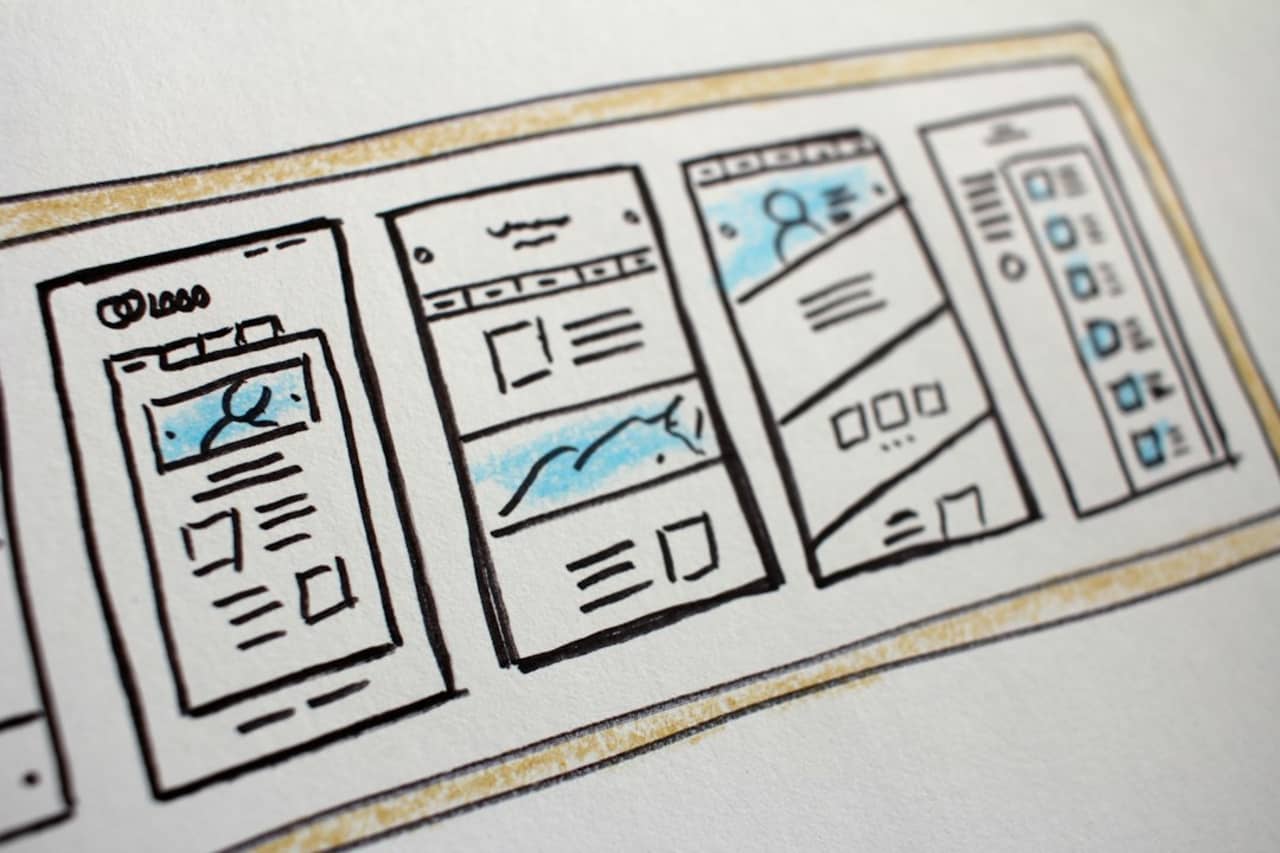
When setting up your Gin project, it’s essential to configure the layout according to your team’s preferences and project requirements. Utilize tools like gin-gonic/gin
, a minimalistic web framework, to streamline routing and middleware management. Additionally, leverage Gin’s support for HTTP handlers, middleware, and routing groups to structure your application efficiently.
When configuring the project layout for your Gin application, consider the following guidelines:
Consistency:
Maintain a consistent directory structure across your projects to facilitate navigation and reduce cognitive overhead for developers.
– Convention over Configuration: Follow established conventions and best practices whenever possible to promote code familiarity and maintainability.
Flexibility: Design your project layout to accommodate future growth and evolution. Anticipate changes in requirements and adapt your structure accordingly to prevent unnecessary refactoring.
Best Practices for Structuring a Gin Project
Follow these best practices to maintain a clean and scalable Gin project:
Modularize Your Application:
- Break down your application into smaller, more manageable modules or packages. Each module should encapsulate a distinct set of functionality and adhere to the single responsibility principle.
- Use Go’s package system to create cohesive and reusable components that can be easily integrated into other projects.
Separate Concerns:
- Adopt a layered architecture that separates concerns such as presentation, business logic, and data access. This separation improves code maintainability, facilitates testing, and enables independent development of different components.
- Utilize design patterns such as MVC (Model-View-Controller) or MVVM (Model-View-ViewModel) to further delineate responsibilities and promote code organization.
Use Dependency Injection:
- Decouple components by injecting their dependencies rather than instantiating them directly. Dependency injection promotes loose coupling and facilitates unit testing by allowing dependencies to be easily mocked or replaced.
- Consider using a dependency injection framework or library, such as Google Wire or Facebook’s Inject, to automate dependency resolution and reduce boilerplate code.
Handle Errors Gracefully:
- Implement robust error handling mechanisms throughout your application to gracefully handle unexpected failures and provide informative error messages to users.
- Use Go’s built-in error handling features, such as the
error
interface and thepanic
andrecover
functions, to manage errors effectively and propagate them up the call stack when necessary.
Document Your Code:
- Write clear and concise documentation for your code using Go’s built-in documentation comments (doc comments). Document the purpose, behavior, and usage of functions, types, and packages to aid comprehension and facilitate collaboration.
- Generate documentation using tools such as
godoc
orpkg.go.dev
to provide developers with easily accessible and up-to-date documentation for your project.
GIN Example:
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
// Initialize Gin router
router := gin.Default()
// Define routes
router.GET("/", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{
"message": "Welcome to Gin Framework!",
})
})
// Start the server
router.Run(":8080")
}
This example demonstrates a basic Gin application with a single route that responds with a JSON message. As your project grows, you can expand this structure to accommodate additional routes, middleware, and business logic.
In conclusion, organizing your Gin project effectively is crucial for its success. By adhering to best practices and maintaining a well-structured codebase, you can enhance the maintainability, scalability, and readability of your Gin applications.